Getting Started with Consumer Data SDK
This SDK helps you to interact with Consumer Data API. Helpful methods include:
- Finding consumer information by passing in a valid phone number
- Simple response object that provides information about the consumer's phone number.
- Helpful extension methods to know if the consumer is blacklisted
In order to get started with Hubtel.ConsumerData.Sdk, do the following activities:
Install-Package Hubtel.ConsumerData.Sdk
This command is run in the Package Manager Console in Visual Studio, and it adds the SDK to your project.
Install-Package Hubtel.ConsumerData.Sdk
Add to appsettings.json
"BearerToken": "<token>"
is used to set the bearer token for authentication. The<token>
placeholder should be replaced with the actual token value."Url": "consumer_data_url"
This key-value pair sets the URL for the consumer data service. Theconsumer_data_url
should be replaced with the actual URL of the service."RedisHost": "localhost"
This key-value pair sets the host for a Redis database. In this case, the host is set tolocalhost
, which means the Redis database is running on the same machine as the application."RedisPort": "<port>"
This key-value pair sets the port number for the Redis database. The<port>
placeholder should be replaced with the actual port number."RedisDbNumber": "<db_number>"
is key-value pair sets the database number for the Redis database. The<db_number>
placeholder should be replaced with the actual database number.
"ConsumerDataConfig": {
"BearerToken": "<token>",
"Url": "consumer_data_url",
"RedisHost": "localhost",
"RedisPort": "<port>",
"RedisDbNumber": "<db_number>"
},
Add to IServiceCollection in Program.cs or Startup.cs
The services.AddHubtelConsumerDataSdk(o => {...})
is a method call that adds theHubtelConsumerDataSdk service
to theIServiceCollection
.IServiceCollection
is an interface in .NET Core that is used for dependency injection. It maintains a collection of service descriptors, which are used to build a service container that provides requested services.Inside the method call, a lambda expression is used to configure the
HubtelConsumerDataSdk
service. Theo
parameter represents the options object for theHubtelConsumerDataSdk
service.The
Url
,BearerToken
,RedisHost
,RedisPort
, andRedisDbNumber
properties of the options object are being set using values from the_configuration
object. The_configuration
object is an instance ofIConfiguration
, which is a standard .NET interface for working with configuration data. It's used to retrieve configuration settings from various sources like environment variables, command-line arguments, or configuration files likeappsettings.json
.The keys used to retrieve the values (like
"ConsumerDataConfig:Url"
) correspond to the keys in the configuration source. For example, inappsettings.json
, you might have aConsumerDataConfig
section withUrl
,BearerToken
,RedisHost
,RedisPort
, andRedisDbNumber
properties.The
GetValue<T>
method is used to retrieve the value of a configuration setting and cast it to a specific type. In this case,RedisHost
is cast to astring
, andRedisPort
andRedisDbNumber
are cast toint
.
services.AddHubtelConsumerDataSdk(o =>
{
o.Url = _configuration["ConsumerDataConfig:Url"];
o.BearerToken = _configuration["ConsumerDataConfig:BearerToken"];
o.RedisHost = _configuration.GetValue<string>("ConsumerDataConfig:RedisHost");
o.RedisPort = _configuration.GetValue<int>("ConsumerDataConfig:RedisPort");
o.RedisDbNumber = _configuration.GetValue<int>("ConsumerDataConfig:RedisDbNumber");
});
Inject and consume service methods
The code below shows the usage of an instance of
IConsumerDataApi
to find account information and perform some operations based on the response.The
private readonly IConsumerDataApi _consumerDataApi
is a of typeIConsumerDataApi
, which is an interface defining methods for interacting with a consumer data API. Theprivate
keyword means this field can only be accessed within the same class. Thereadonly
keyword means that the value of this field can only be assigned at the time of declaration or in the constructor of the class.The line
var response = await _consumerDataApi.FindAccount("<phone_number>")
is calling theFindAccount
method on the_consumerDataApi
instance. TheFindAccount
method takes a phone number as a parameter and returns a task representing the asynchronous operation. Theawait
keyword is used to pause the execution of the method until the awaited task completes. The result of the task is assigned to theresponse variable
. The<phone_number>
is a placeholder and should be replaced with an actual phone number when using this code.The
if (response.IsBlacklisted()) {...}
block checks if the response indicates that the account is blacklisted. If it is, the code inside the block is executed.The
if (response.IsSuccessful) {...}
block checks if the response indicates that the operation was successful. If it was, the code inside the block is executed. Thevar name = response.Data.GetName()
line inside this block retrieves the name from the data in the response.
private readonly IConsumerDataApi _consumerDataApi;
//P.S. be sure to include as constructor parameter
var response = await _consumerDataApi.FindAccount("<phone_number>");
if (response.IsBlacklisted())
{
//your logic here
}
if (response.IsSuccessful)
{
//do something
var name = response.Data.GetName();
}
Was this page helpful?
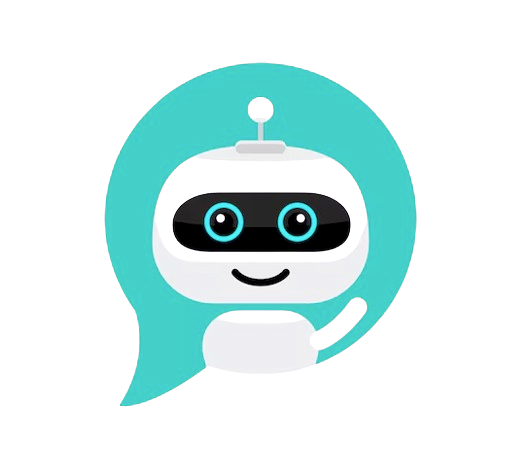
CHAT SAMMIAT