Getting Started with Hubtel File Upload SDK
This SDK helps you to interact with Hubtel File Upload API. Helpful methods include:
- Uploading image in Base64 format
- Uploading image in IFormFile format
- Uploading files in IFormFile format
- Deleting a file using full file path
In order to get started with Hubtel.FileUpload.Sdk, do the following activities:
Install-Package Hubtel.FileUpload.Sdk
The provided command Install-Package Hubtel.FileUpload.Sdk
is used in the NuGet Package Manager Console in Visual Studio. This command is written in PowerShell and it's used to install a specific package into the current .NET project.
Install-Package Hubtel.FileUpload.Sdk
Add to appsettings.json
The FileUploadConfig
object contains several properties:
CloudFrontBaseUrl
: The base URL of the Amazon CloudFront distribution. CloudFront is a content delivery network (CDN) offered by Amazon Web Services (AWS).Bucket
: The name of the S3 bucket where the files will be stored.Folder
: The name of the folder within the S3 bucket where the files will be stored.DefaultSize
: The default size for the uploaded files. This is likely used when resizing images.Sizes
: An array of sizes. These are likely the different sizes to which images will be resized.AccessKey
: The access key for the AWS account.Secret
: The secret key for the AWS account.FileUploadApiBaseUrl
: The base URL of the file upload API.AwsS3BaseUrl
: The base URL of the Amazon S3 service.UseCustomS3Config
: A boolean indicating whether a custom S3 configuration should be used.AuthToken
: The authentication token used when making requests to the file upload API.
This configuration allows the application to connect to the file upload API, authenticate using the provided token, and interact with the S3 bucket. It also specifies the sizes for resizing images and whether a custom S3 configuration should be used.
{
"FileUploadConfig": {
"CloudFrontBaseUrl": "<cloud_front_base_url>",
"Bucket": "<bucket>",
"Folder": "<folder>",
"DefaultSize": "720x720",
"Sizes": [
"256x256",
"512x512"
],
"AccessKey": "<access_key>",
"Secret": "<secret>",
"FileUploadApiBaseUrl": "http://localhost:5001",
"AwsS3BaseUrl": "<aws_base_url>",
"UseCustomS3Config": true,
"AuthToken": "PrivateKey <token>"
}
}
Notes
DefaultSize
: This is the default value to be used to resize an image.Size
: This will be used to resize an image into other sizes apart from the default size- You can ignore DefaultSize and Sizes if you won't be doing image uploads
AccessKey
,Secret
,AwsS3BaseUrl
,CloudFrontBaseUrl
can be ignored when using the same config as as the File Upload API. If you'll be using same config as that of the File Upload API, setUseCustomS3Config
tofalse
.AccessKey
,Secret
,AwsS3BaseUrl
,CloudFrontBaseUrl
are test configs. DO NOT use them on production
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelFileUploadSdk(x => Configuration.GetSection(nameof(FileUploadConfig)).Bind(x)
is adding the Hubtel File Upload SDK to the service collection. This makes the SDK available to be injected into other parts of the application where it's needed.The
AddHubtelFileUploadSdk
method is a custom extension method that likely sets up the necessary services for the SDK. It takes a lambda function as a parameter, which is used to configure the SDK.The lambda function
x => Configuration.GetSection(nameof(FileUploadConfig)).Bind(x)
is retrieving theFileUploadConfig
section from the application's configuration (usually defined inappsettings.json
or another configuration source), and binding it to the SDK's configuration object. This allows the SDK to be configured based on the settings defined in theFileUploadConfig
section.
services.AddHubtelFileUploadSdk(x => Configuration.GetSection(nameof(FileUploadConfig)).Bind(x));
Inject and consume service methods
private readonly IUploadService _uploadService
;
- This line declares a private, read-only field named
_uploadService
of typeIUploadService
. This is presumably an interface that defines methods for uploading and deleting files. The comment suggests that an instance ofIUploadService
should be provided as a constructor parameter.
The following lines of code demonstrate how to use the _uploadService
to upload and delete files:
var response = await _uploadService.UploadBase64Image(base64ImageString);
- This line uploads an image that is encoded as a Base64 string. The response from the upload operation is stored in the
response
variable.
var response = await _uploadService.UploadImageFile(imageIFormFile);
- This line uploads an image that is provided as an IFormFile, which is a .NET interface representing a file sent with the HttpRequest.
var response = await _uploadService.UploadFile(iFormFile);
- This line uploads a file that is provided as an
IFormFile
.
var response = await _uploadService.DeleteFile("250x250/testfolder/1ddb1a9df17c467f813696fbef940adf.jpg");
- This line deletes a file at the specified path. The response from the delete operation is stored in the
response
variable.
private readonly IUploadService _uploadService;
//P.S. be sure to include as constructor parameters
// Upload image in base64 format
var response = await _uploadService.UploadBase64Image(base64ImageString);
// Upload image in iformfile format
var response = await _uploadService.UploadImageFile(imageIFormFile);
// Upload file in iformfile format
var response = await _uploadService.UploadFile(iFormFile);
// Deleting a file using full file path
var response = await _uploadService.DeleteFile("250x250/testfolder/1ddb1a9df17c467f813696fbef940adf.jpg");
Was this page helpful?
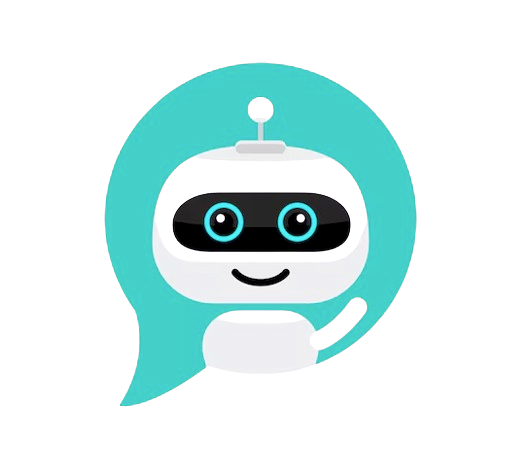
CHAT SAMMIAT