Core Identity.
Core Identity is a Flutter package designed to streamline the integration of facial verification into Hubtel’s Flutter applications. By utilizing the Core Identity package, developers can easily incorporate robust liveness detection capabilities into their Flutter apps, enhancing security and user experience.
Installation
To get started with Core Identity, add the dependency to your pubspec.yaml
core_identity:
git:
url: git@ssh.dev.azure.com:v3/hubtel/Mobile-Apps/Platform-Library-Flutter-Core-Identity
ref: 1.0.32 //Use latest version
Usage
Call CoreIdentity.startVerification
to initiate the verification flow.
Params
context
(BuildContext): The current widget tree's context.foregroundColor
(Color?, optional): Customizes the foreground color of the verification UI.backgroundColor
(Color?, optional): Customizes the background color of the verification UI.ghanaCardNumber
(String?, optional): The user's Ghana Card number, if available.phoneNumber
(String, required): The phone number to be verified.analytics
(IAnalyticsUtils, required): An analytics utility object for tracking the verification process.
Return Value
The function returns a Future<VerificationResult?>
. This future resolves to:
A
VerificationResult
object if the verification process completes successfully.VerificationResult
contains:id
: User's identification (e.g., Ghana Card Number)status
: Enum indicating success, failure or idle.idle
- Verification has not yet startedfailure
- Verification failed or cancelledsuccess
- User verified successfully.
image
: Verification imagemessage
: Empty string on success, failure reason otherwise
null
if the verification process is cancelled or fails.
Exceptions
The function will throw an AssertionError
if the provided phoneNumber
is blank.
Example Usage
final verificationResult = await CoreIdentity.startVerification(
context,
phoneNumber: result?.mobileNumber ?? "",
analytics: HubtelLibraryAnalyticsUtils.instance,
);
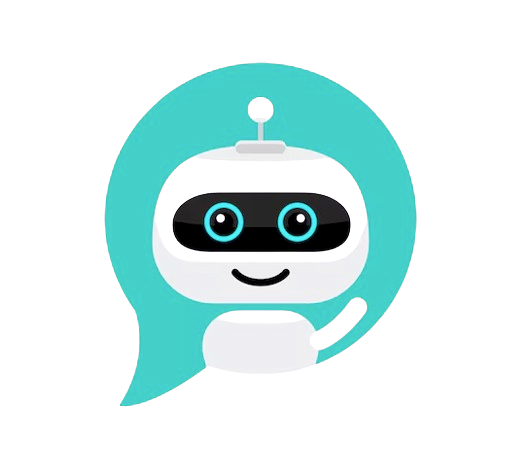
CHAT SAMMIAT