Rest API Tests in Flutter Apps
Writing tests, including API tests, is integral to ensuring the reliability and stability of a Flutter application. Flutter offers a comprehensive testing framework that enables developers to create unit tests, widget tests, and integration tests. Specifically, in the context of Hubtel, HTTP packages like http
are commonly employed to facilitate communication with APIs. It's important to note that API testing typically falls within the category of unit tests.
Unit Testing in Flutter
A unit test in Flutter is designed to evaluate the correctness of a single function, method, or class. The primary objective is to validate the behavior of a discrete unit of logic across various scenarios.
Sample Api test from one of our apps
void main() {
late TestApiRequester testApiRequester;
late InstantServiceApi instantServiceApi;
setUp(() {
testApiRequester =
TestApiRequester(testProfile: DefaultTestProfileSet.testProfile1);
instantServiceApi = InstantServiceApi(requester: testApiRequester);
});
group("Send Money Test", () {
test("Get Send Money History", () async {
var result = await instantServiceApi.getServiceHistory(
serviceType: InstantServicesStrings.categoryType,
req: GetQueryPagedReq(page: 0, query: ""));
expect(result.response?.status, ApiResponseStatus.Success);
});
});
}
Let's break down the construct used:
Test Construct Breakdown
setUp
The setUp function is a special function that runs before each test case within the group. It is typically used to set up any necessary resources or initialize objects that are required for the tests. It's used to initialize objects and set up resources before each test case within the group.
setUp(() {
testApiRequester =
TestApiRequester(testProfile: DefaultTestProfileSet.testProfile1);
instantServiceApi = InstantServiceApi(requester: testApiRequester);
});
Here, before each test inside the Send Money Test
group, we are creating instances of TestApiRequester and InstantServiceApi using a specific test profile.
group
The group
function is used to organize tests into logical groups. It helps in structuring your test suite, making it easier to understand and manage.
group("Send Money Test", () {
// Test cases related to sending money
});
This creates a group named Send Money Test
and contains one or more test cases related to sending money.
test
The test function is used to define an individual test case. It takes a description of the test case and a callback function that contains the actual test logic.
test("Get Send Money History", () async {
var result = await instantServiceApi.getServiceHistory(
serviceType: InstantServicesStrings.categoryType,
req: GetQueryPagedReq(page: 0, query: ""));
expect(result.response?.status, ApiResponseStatus.Success);
});
This test case is named Get Send Money History
and it calls the getServiceHistory
method on instantServiceApi
. The expect function is then used to assert that the status of the response is ApiResponseStatus.Success
.
expect and equals
The expect function is used to assert that a given condition is true. If the condition is false, the test fails. It is commonly used with the equals matcher to check if two values are equal.
expect(result.response?.status, ApiResponseStatus.Success);
Here, you're expecting that the status of the response is equal to ApiResponseStatus.Success
.
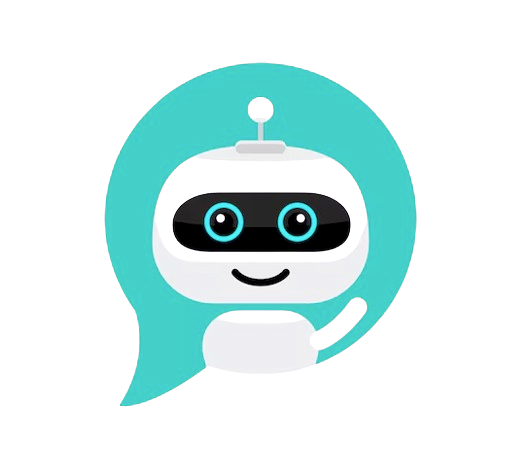
CHAT SAMMIAT