Unified Checkout SDK
The Hubtel Flutter Checkout Package is a convenient and easy-to-use library that simplifies the process of implementing a checkout flow in your Flutter application. This sdk is for hubtel internal merchants. Please read the docs instructions below to be able to integrate successfully.
NB: Before calling the Checkout SDK, most internal apps would call a Pre-Checkout API provided by their backend team.
Sample Pre-checkout Request
{
"sessionId": "b91955dd596c4bd28986e77c2ae95b58",
"serviceId": "3e0841e70afc42fb97d13d19abd36384",
"integrationChannel": "mobile-android"
}
Sample Pre-checkout Response
{
"message": "success",
"code": "200",
"data": {
"description": "Payment of GHS 1.00 for (18013782) (MR SOMUAH STA ADANE-233557913587) ",
"clientReference": "f03dbdcf8d4040dd88d0a82794b0229f",
"amount": 1.00,
"customerMobileNumber": "233557913587",
"callbackUrl": "https://instantservicesproxy.hubtel.com/v2.1/checkout/callback",
"merchantApiKey": "gfjgfkuastgfs433ug35g454",
"merchantId": 554653
}
}
The response from the Pre-Checkout API usually contains all information needed for a successful checkout. Once retrieved the data is then passed onto the Hubtel Checkout SDK to begin the checkout. Follow the link below for more information on the Hubtel Checkout SDK. External Merchant Checkout
Features
- Direct Debit feature: A feature to debit users on first approval, and subsequent ones without asking for pin.
- Pre approval confirm: A feature where amount can be debited later when service is rendered.
- Receive money prompt: A feature that works like the normal momo where authorization is needed before debit
- Bank Pay: A feature where user downloads a payment receipt to make payments in a bank.
- Zeepay: Make payment with zeepay
- Gmoney: Make payment with gmoney
- Hubtel: Make payment with your hubtel wallet
Getting started
To install the Internal Checkout SDK, add the code below to your pubspec.yaml.
NB: ref represents the version number and is subjected to change.
checkout_sdk_internal:
git:
url: [email protected]:v3/hubtel/Mobile-Apps/Platform-Library-Flutter-Checkout-SDK
ref: 1.0.6
Usage
_checkoutInternal({required BuildContext context}) async {
final onCheckoutDone = await Navigator.push(
context,
MaterialPageRoute(
builder: (context) {
return CheckoutSDK.getCheckoutScreen(
purchaseInfo: PurchaseInfo(
amount: 1,
customerPhoneNumber: "233245045867",
purchaseDescription: "here",
clientReference: Uuid().v4(),
// deliveryFee: 1,
extras:"Base 64 encoded service details"
),
config: CheckoutConfiguration(
branchId: "6ba88b25677f4daa9907d04cb1cea00f",
callbackUrl:
'https://b0ed-154-160-4-43.ngrok-free.app/payment/callback',
businessId: "ericacormey",
bearerToken:"Bearer Token"
),
analyticsData: CheckoutAnalyticsData(
appName: "Checkout Test App",
appCategory: "Test",
),
);
},
),
);
if (onCheckoutDone is CheckoutCompletionStatus) {
print(onCheckoutDone.status);
}
}
Integration in the Hubtel App
Note: For the Hubtel App, the Hubtel Merchant SDK will be abstracted, which means all calls to checkout would have to go through the abstraction. More details will be shared here, when available.
To use Unified Checkout on the Hubtel App, simply call Checkout.Navigation().gotoUnifiedCheckout(...some args)
Argument definition for Checkout.Navigation().gotoUnifiedCheckout()
Argument: Type | Description |
---|---|
unifiedCheckoutConfig: UnifiedCheckoutConfig | Configuration for a checkout. This contains all details needed for a successful checkout. |
context: BuildContext | The context of the current widget. |
onCheckoutCompleted(response: UnifiedCheckoutResponse): ValueChanged<UnifiedCheckoutResponse> | A callback that is called when the checkout is completed. The response contains the status of the checkout. |
UnifiedCheckoutConfig
class UnifiedCheckoutConfig {
final double amount;
final String callbackUrl;
final String merchantApiKey;
final String merchantId;
final String? clientReference;
final String? customerMobileNumber;
final String? description;
UnifiedCheckoutConfig({
required this.amount,
required this.callbackUrl,
required this.merchantApiKey,
required this.merchantId,
this.clientReference,
this.customerMobileNumber,
this.description,
});
}
UnifiedCheckoutResponse
class UnifiedCheckoutResponse {
final String transactionId;
final PaymentStatus status;
UnifiedCheckoutResponse({
required this.transactionId,
required this.status,
});
}
enum PaymentStatus {
unpaid,
paid,
pending,
unspecified,
}
Usage of Checkout.Navigation().gotoUnifiedCheckout
The code below shows a sample implementation of Unified Checkout for Airtime Data and Bills.
final preCheckoutResult = await adbViewModel.preCheckout();
if (!mounted) return;
if (preCheckoutResult.state == UiState.success && preCheckoutResult.data != null) {
Checkout.Navigation().gotoUnifiedCheckout(
context,
args: preCheckoutResult.data!.toUnifiedCheckoutConfig(),
onCheckoutCompleted: (response) {
adbViewModel.resetFinishSessionUiState();
if (response.status == UnifiedCheckoutStatus.success) {
adbViewModel.resetPurchaseServiceState();
Navigation.openTransactionStatusScreen(context)
}
},
);
}
Models
CheckoutConfiguration
This encapsulates information about the purchase.
Parameter: Type | Description |
---|---|
branchId: String | The branchId of the business. |
callbackUrl: String | Payment results will be sent to this callback Url |
businessId: String | The business id of the purchase. |
bearerToken | The token of the user making the payment. |
PurchaseInfo
This encapsulates configuration information for the checkout.
Parameter: Type | Description |
---|---|
amount: Double | The amount the customer is paying |
customerPhoneNumber: String | The number of the customer making payment |
purchaseDescription: String | The description of the purchase |
clientReference | The client reference of the purchase. |
extras | base 64 encoded version of the service details. |
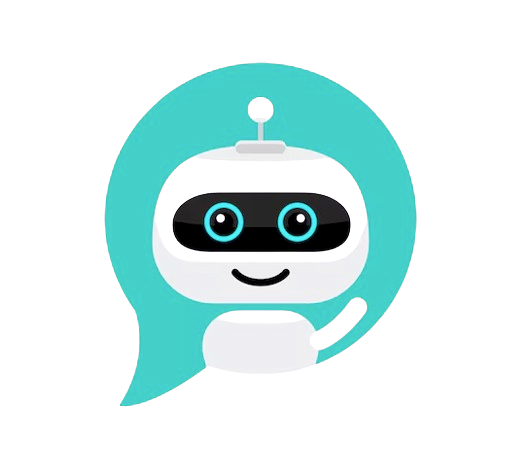
CHAT SAMMIAT