Getting Started with Hubtel AirtelTigo SDK
This SDK helps you to interact with the AirtelTigo API. Helpful methods include:
- Perform a name query using a valid AirtelTigo Number (Validate an AirtelTigo account)
In order to get started with Hubtel.AirtelTigo.Sdk, do the following activities:
Install-Package Hubtel.AirtelTigo.Sdk
The code snippet beneath is a command that is used to install Hubtel.AirtelTigo.Sdk
package in a .NET project.
Install-Package Hubtel.AirtelTigo.Sdk
Add to appsettings.json
The HubtelAirtelApiConfig
object contains several properties that are used to configure the Hubtel Airtel API integration:
Url
: This is the URL of the Hubtel Airtel API endpoint. You would replace<url>
with the actual URL.Username
: This is the username used for authentication with the Hubtel Airtel API. You would replace<username>
with your actual username.Password
: This is the password used for authentication with the Hubtel Airtel API. You would replace<password>
with your actual password.TimeOut
: This is the timeout value for requests to the Hubtel Airtel API. You would replace<timeout>
with the actual timeout value.AuthScheme
: This is the authentication scheme used for the Hubtel Airtel API. In this case, it's set to "bearer", which indicates that bearer token authentication is used.Token
: This is the bearer token used for authentication with the Hubtel Airtel API. In this case, it's a placeholder string "bearer token here". In a real application, this would be replaced with the actual bearer token.
These values are typically sensitive and should not be hard-coded into your application's source code. Instead, they should be stored securely and retrieved from the configuration at runtime.
"HubtelAirtelApiConfig": {
"Url":"<url>",
"Username": "<username>",
"Password": "<password>",
"TimeOut": "<timeout>",
"AuthScheme": "bearer",
"Token": "bearer token here"
}
Add to IServiceCollection in Program.cs or Startup.cs
The AddHubtelAirtelTigoSdk
method takes a lambda expression as an argument, which is used to configure the options for the HubtelAirtelTigoSdk
service. The option
parameter in the lambda expression is an object that has properties corresponding to the configuration options for the service.
Each property of the option
object is being set to a value from the application's configuration, which is accessed through the hostContext.Configuration
object:
option.Url
is set to theUrl
value in theHubtelAirtelApiConfig
section of the configuration.option.Username
is set to theUsername
value in theHubtelAirtelApiConfig
section of the configuration.option.Password
is set to thePassword
value in theHubtelAirtelApiConfig
section of the configuration.option.Timeout
is set to theTimeOut
value in theHubtelAirtelApiConfig
section of the configuration. Theint.Parse
method is used to convert the string value from the configuration to an integer.option.AuthScheme
is set to theAuthScheme
value in theHubtelAirtelApiConfig
section of the configuration.option.Token
is set to theToken
value in theHubtelAirtelApiConfig
section of the configuration.
services.AddHubtelAirtelTigoSdk(option =>
{
option.Url = hostContext.Configuration["HubtelAirtelApiConfig:Url"];
option.Username = hostContext.Configuration["HubtelAirtelApiConfig:Username"];
option.Password = hostContext.Configuration["HubtelAirtelApiConfig:Password"];
option.Timeout = int.Parse(hostContext.Configuration["HubtelAirtelApiConfig:TimeOut"]);
option.AuthScheme = hostContext.Configuration["HubtelAirtelApiConfig:AuthScheme"];
option.Token = hostContext.Configuration["HubtelAirtelApiConfig:Token"];
});
Inject and consume service methods
The IAirtelTigoApi
interface is injected into the class via the constructor (as indicated by the comment), and is stored in the _airtelTigoApi
field.
The code then shows how to use four methods of the IAirtelTigoApi
interface:
ValidateUserAccountAsync("<validMsiSdn>")
: This method is used to validate an AirtelTigo account using a valid MSISDN (Mobile Station International Subscriber Directory Number, a unique identifier for a mobile phone user). The method is asynchronous and returns a Task that, when awaited, provides the result of the validation.SendMoneyAsync(new SendMoneyRequest {...})
: This method is used to send money to an AirtelTigo account. It takes a SendMoneyRequest object as a parameter, which includes details of the transaction such as the recipient's MSISDN, the amount to send, an external transaction ID, a reference, and a reference number.ReceiveMoneyAsync(new ReceiveMoneyRequest {...})
: This method is used to receive money from an AirtelTigo account. It takes a ReceiveMoneyRequest object as a parameter, which includes details of the transaction such as the customer's MSISDN, the amount to receive, a reference, and a business name.CheckTransactionStatusAsync("<transaction_id>")
: This method is used to check the status of a transaction. It takes a transaction ID as a parameter.
In each case, the result of the operation is stored in the result
variable. Note that these methods are all asynchronous, so they are awaited using the await
keyword. This means that the method in which this code is running must be an asynchronous method itself.
private readonly IAirtelTigoApi _airtelTigoApi;
//P.S. be sure to include as constructor parameters
// Perform a name query using a valid AirtelTigo Number (Validate an AirtelTigo account)
var result = await _airtelTigoApi.ValidateUserAccountAsync("<validMsiSdn>");
// Perform a send money transaction
var result = await _airtelTigoApi.SendMoneyAsync(new SendMoneyRequest
{
MsiSdn = "<validMsiSdn>",
Amount = 1.00,
ExternalTransactionId = "<external_transaction_id>",
Reference = "<reference>",
ReferenceNumber = "<reference_number>"
});
// Perform a receive money transacation
var result = await _airtelTigoApi.ReceiveMoneyAsync(new ReceiveMoneyRequest
{
CustomerMsisdn = "<validMsiSdn>",
Amount = 5,
Reference = "<reference>",
BusinessName = "<business_name>"
});
// Perform a check transaction status
var result = await _airtelTigoApi.CheckTransactionStatusAsync("<transaction_id>");
Was this page helpful?
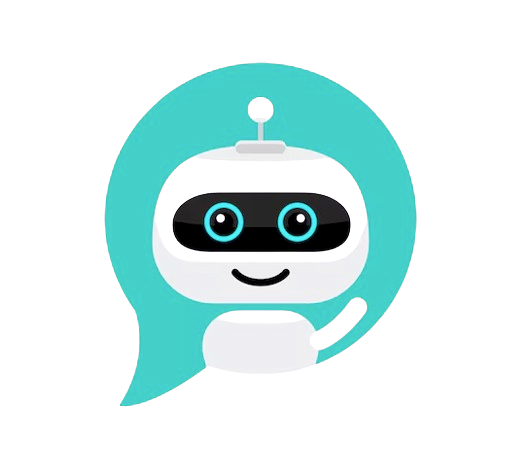
CHAT SAMMIAT