Core Storage Library Documentation
Overview
The Core Storage Library provides a robust set of tools for managing local storage, database operations, and feature release management in Flutter applications. It offers abstractions for shared preferences, SQLite database interactions, and Firebase Remote Config integration.
Key Components
1. Base Preference Manager
The BasePreferenceManager
class provides a comprehensive interface for interacting with SharedPreferences. It includes methods for saving and retrieving various data types, including strings, booleans, integers, doubles, and complex objects.
2. Database Manager
The DataBaseManagerBase
class offers an abstraction layer for SQLite database operations using the sqflite
package.
Key features:
- Create and manage SQLite databases
- Execute table creation statements
- Insert, retrieve, and delete records
- Perform batch operations
3. Feature Release Manager
The Feature Release Manager component provides a mechanism for managing feature flags and rollouts using Firebase Remote Config.
Key features:
- Define feature configurations
- Check if a feature is enabled for specific users or stations
- Integrate with Firebase Remote Config for dynamic feature management
4. Remote Config
The BaseRemoteConfig
class offers a wrapper around Firebase Remote Config, providing easy access to remote configuration values.
Key features:
- Initialize and configure Firebase Remote Config
- Fetch and parse configuration values as maps
Usage
Shared Preferences
To use the BasePreferenceManager:
- Create a class that extends
BasePreferenceManager
. - Use the provided methods to save and retrieve data:
class MyPreferenceManager extends BasePreferenceManager {
Future<void> saveUsername(String username) async {
saveToSharedPref('username', username);
}
Future<String> getUsername() async {
return await getSharedPrefString('username');
}
}
Database Operations
To use the DatabaseManagerBase:
- Create a class that extends
DataBaseManagerBase
. - Implement methods for your specific database operations:
class MyDatabaseManager extends DataBaseManagerBase {
Future<Database> initializeDatabase() async {
return await createDataBase(
dbPath: 'my_database.db',
statement: 'CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)',
);
}
Future<void> insertUser(String name) async {
final db = await initializeDatabase();
await insertIntoDb(
values: {'name': name},
db: db,
dbName: 'users',
);
}
}
Feature Release Management
To use the Feature Release Manager:
- Initialize the FeatureReleaseManager:
await FeatureReleaseManager.init();
- Check if a feature is enabled:
final featureManager = FeatureReleaseManager(MyAppSection());
bool isEnabled = await featureManager.isFeatureEnabled('my_feature_key');
Best Practices
- Use the BasePreferenceManager for storing small amounts of data or application settings.
- Leverage the DatabaseManagerBase for more complex data structures or when you need to perform queries.
- Utilize the Feature Release Manager for gradual rollouts of new features or A/B testing.
- Keep sensitive information encrypted when storing in SharedPreferences or the local database.
- Regularly sync Remote Config to ensure your app has the latest feature flags and configurations.
Conclusion
The Core Storage Library provides a comprehensive set of tools for managing local data persistence, database operations, and feature releases in Flutter applications. By leveraging these components, developers can efficiently handle data storage needs and implement dynamic feature management in their apps.
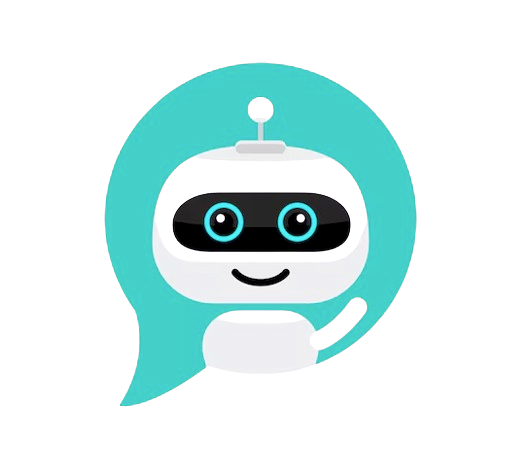
CHAT SAMMIAT