Getting Started with Accounting SDK
An accounting SDK is a set of tools, libraries, and APIs (Application Programming Interfaces) provided by a software company to enable developers to integrate accounting functionality into their own applications. Essentially, it's a package of resources that simplifies the process of incorporating accounting features into software products.
Accounting SDKs typically offer a range of functionalities related to financial management, such as invoicing, expense tracking, ledger management, reporting, and integration with accounting systems like QuickBooks, Xero, or FreshBooks. By using an accounting SDK, developers can leverage pre-built components and APIs to accelerate development and ensure compatibility with established accounting standards and practices.
These SDKs often include documentation, code samples, and technical support to assist developers in integrating accounting features seamlessly into their applications. Additionally, they may offer customization options to tailor the accounting functionality to specific business needs or industries.
In order to get started with Hubtel.Accounting.Sdk, do the following activities:
Install-Package Hubtel.Accounting.Sdk
This command is used to install a NuGet package into your project. NuGet is a package manager for the Microsoft development platform, and it's a way to distribute and consume reusable code. Hubtel.Accounting.Sdk
is the name of the package to be installed.
Install-Package Hubtel.Accounting.Sdk -version 8.0.1
Add to IServiceCollection in Program.cs or Startup.cs
In your Startup.cs
or Program.cs
file, you would add the Accounting SDK service to the IServiceCollection
:
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddAccountingSdk(options =>
{
options.ApiKey = Configuration["AccountingSdk:ApiKey"];
options.Secret = Configuration["AccountingSdk:Secret"];
});
}
}
Add to appsettings.json
AccountingSdk
is a configuration section that contains settings for the Accounting SDK.
{
"AccountingSdk": {
"ApiKey": "your-api-key",
"Secret": "your-secret-key"
}
}
Inject and consume service methods
In a controller or other class where you want to use the service, you would inject the service into the constructor and assign it to a private field:
public class AccountingController : Controller
{
private readonly IAccountingService _accountingService;
public AccountingController(IAccountingService accountingService)
{
_accountingService = accountingService;
}
public async Task<IActionResult> Index()
{
var invoices = await _accountingService.GetInvoices();
return View(invoices);
}
}
IAccountingService
is a hypothetical interface provided by the Accounting SDK. You would replace IAccountingService
with the actual interface or class provided by your specific Accounting SDK.
Finally, you would use the _accountingService
field to call methods provided by the Accounting SDK:
public async Task<IActionResult> CreateInvoice(InvoiceModel model)
{
var invoice = await _accountingService.CreateInvoice(model);
return View(invoice);
}
Was this page helpful?
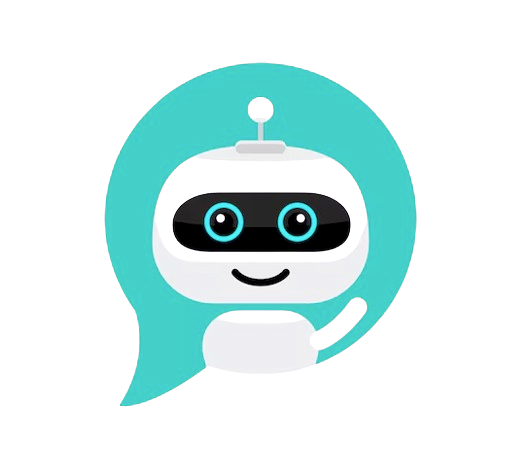
CHAT SAMMIAT