Getting Started with Polly
Polly Validation is a .NET library which helps implement resilience and fault handling policies to make your application more robust and resilient when interacting with external systems. By providing policies such as Retry, WaitAndRetry, and CircuitBreaker, Polly can help you reduce fragility, and keep your systems and customers connected.
In order to get started with Polly, do the following activities
Installing Polly Package
Install-Package Polly
Configuration
After installing the Polly package, configure and apply policies to your services. These are used to define behaviors for handling faults, retries, and circuit breaking when making HTTP requests.
//Configure WaitAndRetry policy
_retryPolicy = Policy.Handle<HttpRequestException>()
.OrResult<HttpResponseMessage>(r => !r.IsSuccessStatusCode)
.WaitAndRetryAsync(3, retryAttempt => TimeSpan.FromSeconds(Math.Pow(2, retryAttempt)));
//Configure Circuit Breaker policy
_circuitBreakerPolicy = Policy.Handle<Exception>()
.CircuitBreakerAsync(
exceptionsAllowedBeforeBreaking: 3,
durationOfBreak: TimeSpan.FromMinutes(1)
);
//Configure Timeout policy
_timeoutPolicy = Policy.TimeoutAsync(TimeSpan.FromSeconds(30));
//Configure Fallback policy
_fallbackPolicy = Policy<string>.Handle<Exception>()
.FallbackAsync("Fallback value");
//Configure Bulkhead policy
_bulkheadPolicy = Policy.BulkheadAsync(10, 2);
Sample Usage
This is a sample use case with the Retry policy to see how it works.
Configure and apply WaitAndRetry policy to service method
public class MyService
{
private readonly HttpClient _httpClient;
private readonly Policy _retryPolicy;
public MyService()
{
_httpClient = new HttpClient();
// Configure WaitAndRetry policy
_retryPolicy = Policy.Handle<HttpRequestException>()//define the exception or result to handle
.OrResult<HttpResponseMessage>(r => !r.IsSuccessStatusCode)//include non-successful HTTP responses for retrying
.WaitAndRetryAsync(3, retryAttempt => TimeSpan.FromSeconds(Math.Pow(2, retryAttempt)));//specify the retry count and the duration between retries.
}
public async Task<HttpResponseMessage> MakeHttpRequest()
{
return await _retryPolicy.ExecuteAsync(() => _httpClient.GetAsync("https://api.example.com"));//applies the WaitAndRetry policy
}
}
Inject and consume service method
public class MyApplication
{
private readonly MyService _myService;
public MyApp(MyService myService)
{
_myService = myService;
}
public async Task PerformHttpRequest()
{
try
{
HttpResponseMessage response = await _myService.MakeHttpRequest();//HTTP request is performed using WaitAndRetry policy
// Process the response
}
catch (Exception ex)
{
// Handle the exception or log the error
}
}
}
Polly provides various other policies such as Circuit Breaker, Timeout, Bulkhead, etc., which can be configured and applied as needed.
Further Readings
Follow this link to learn more.
Was this helpful? 📚
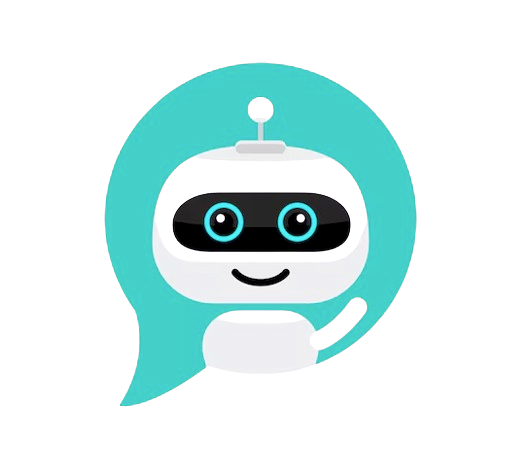
CHAT SAMMIAT