Getting Started with Vodafone SDK
This SDK helps you to perform NameCheck/KYC operations on Vodafone Mobile Money.
In order to get started with Hubtel.Vodafone.Sdk, do the following activities:
Install-Package Hubtel.Vodafone.Sdk
The code snippet below is a command that is used to install Hubtel.Vodafone.Sdk
package in a .NET project.
Install-Package Hubtel.Vodafone.Sdk
Add to IServiceCollection in Program.cs or Startup.cs
services.Configure<VodafoneSettings>(c => Configuration.GetSection(nameof(VodafoneSettings)).Bind(c))
This line is configuring the settings for the Vodafone SDK. It uses the
Configuration
object'sGetSection
method to retrieve theVodafoneSettings
section from the application's configuration (usually found inappsettings.json
). Thenameof
operator is used to get the string name of theVodafoneSettings
section in a type-safe manner.The
Bind
method is then called on the configuration section, binding the configuration values to an instance ofVodafoneSettings (c
in the lambda expression). This means that theVodafoneSettings
object will be populated with the settings from theVodafoneSettings
section of the application's configuration.
services.AddHubtelVodafoneSdk()
- This line is adding the Hubtel Vodafone SDK to the application's services. This is a method provided by the
Hubtel.Vodafone.Sdk
package that sets up any necessary services for the SDK to function, such as HTTP clients or other dependencies.
services.Configure<VodafoneSettings>(c =>
Configuration.GetSection(nameof(VodafoneSettings)).Bind(c));
services.AddHubtelVodafoneSdk();
Inject and consume service methods
private readonly IVodafoneApi _api;
private readonly IVodafoneApiResponseParser _apiResponseParser;
- These lines declare two private, read-only fields.
_api
is of typeIVodafoneApi
, which is an interface provided by the SDK for interacting with the Vodafone API._apiResponseParser
is of typeIVodafoneApiResponseParser
, which is an interface provided by the SDK for parsing responses from the Vodafone API.
var res = await _vodafoneApi.ValidateAccount("0500390299");
- This line calls the
ValidateAccount
method of the_vodafoneApi
object, passing in a string that appears to be a phone number. TheValidateAccount
method is asynchronous, so it returns a task that is awaited using theawait
keyword. The result of the task is assigned to theres
variable.
var res = _apiResponseParser.ParseCallbackResponsePayload(callback)
- This line calls the
ParseCallbackResponsePayload
method of the_apiResponseParser
object, passing in a variable namedcallback
. The method parses a callback response from the Vodafone API and returns the result, which is assigned to theres
variable.
private readonly IVodafoneApi _api;
private readonly IVodafoneApiResponseParser _apiResponseParser;
//P.S. be sure to include as constructor parameter
//doing name check
var res = await _vodafoneApi.ValidateAccount("0500390299");
// parse a callback response xml
var res = _apiResponseParser.ParseCallbackResponsePayload(callback);
Sample VodafoneSettings in appsetings.json
The "VodafoneSettings"
block contains several properties:
"thirdPartyId"
: This property specifies the third-party ID, which is likely used for identification when interacting with the Vodafone API."password"
: This property specifies the password used for authentication with the Vodafone API."shortCode"
: This property specifies the short code, which is typically a unique identifier for a particular service or application."keyOwner"
: This property specifies the key owner, which could be a reference to the owner of an API key or similar credential."pin"
: This property specifies a PIN, which is likely another form of authentication."callbackUrl"
: This property is a placeholder for the URL where Vodafone API will send callback responses."timeout"
: This property specifies the timeout duration in seconds for API requests."kycUrl"
: This property is a placeholder for the URL of the Know Your Customer (KYC) service."kycCallerType"
,"kycInitiatorIDType"
,"kycInitiatorID"
,"kycReceiverPartyIDType"
,"kycCommandID"
: These properties are specific to the KYC service and likely specify various parameters for KYC requests.
"VodafoneSettings": {
"thirdPartyId": "SMSGH",
"password": "82E35",
"shortCode": "749000",
"keyOwner": "1",
"pin": "3745",
"callbackUrl": "<call_back_url>",
"timeout": 60,
"kycUrl": "<kyc_url>",
"kycCallerType": "2",
"kycInitiatorIDType": "12",
"kycInitiatorID": "9495",
"kycReceiverPartyIDType": "1",
"kycCommandID": "QueryCustomerKYC"
}
Was this helpful? 📚
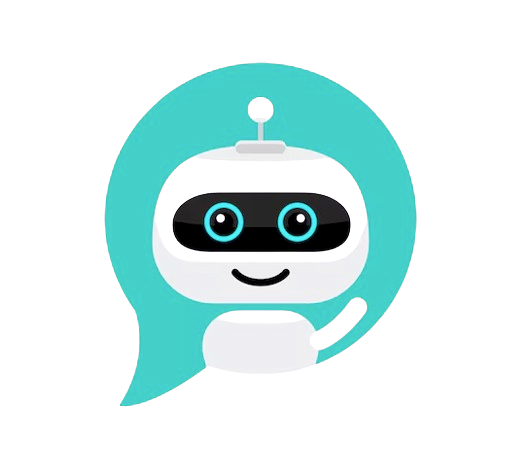
CHAT SAMMIAT