.NET Observability with OpenTelemetry
Observability in distributed systems enables monitoring and analyzing telemetry data—such as logs, metrics, and traces—to assess application performance and diagnose issues. Unlike invasive debugging, observability operates transparently with minimal performance impact, allowing continuous monitoring.
The Three Telemetry Signals of OpenTelemetry
- Logs: Record individual operations, including incoming requests, component failures, or specific events like order placements.
- Metrics: Provide quantitative measurements such as the number of completed requests, active sessions, items sold, or request latency histograms.
- Distributed Tracing: Track requests and activities across components in a distributed system, helping identify performance bottlenecks and trace failures.
These pillars encompass telemetry data from various sources:
- .NET Runtime: Data from the garbage collector or JIT compiler.
- Libraries: Telemetry from components like Kestrel (ASP.NET web server), HttpClient,Kafka , Redis, etc.
- Application Code: Custom telemetry emitted by your application.
Approaches to Implementing Observability in .NET
- Explicit Instrumentation: Incorporate libraries like OpenTelemetry directly into your code. This method offers extensive configurability but requires access to and modification of the source code.
- Out-of-Process Monitoring: Utilize tools such as
dotnet-monitor
andnew-relic-infra-agent
that leverage EventPipe and other system services to capture logs and metrics without altering application code. - Startup Hooks: Inject assemblies into the process at startup to collect instrumentation, exemplified by OpenTelemetry .NET Automatic Instrumentation.
Introduction to OpenTelemetry
OpenTelemetry (OTel) is a cross-platform, open standard for collecting and emitting telemetry data. It comprises:
- APIs for Libraries: Enable recording of telemetry data during code execution.
- APIs for Application Developers: Configure data transmission, including filtering, buffering, enrichment, and transformation.
- Semantic Conventions: Standardize naming and content of telemetry data to ensure consistency between data producers and analysis tools.
- Exporters Interface: Plugins that transmit telemetry data in specific formats to various backends.
- OTLP Wire Protocol: A vendor-neutral protocol for transmitting telemetry data.
By adopting OTel, you can integrate with numerous Application Performance Monitoring (APM) systems, including open-source solutions like Prometheus and Grafana, Azure Monitor, and offerings from various APM vendors.
OpenTelemetry in .NET
The .NET implementation of OpenTelemetry leverages existing framework APIs:
- Logging:
Microsoft.Extensions.Logging.ILogger<TCategoryName>
- Metrics:
System.Diagnostics.Metrics.Meter
- Distributed Tracing:
System.Diagnostics.ActivitySource
andSystem.Diagnostics.Activity
OpenTelemetry collects telemetry from these APIs and exports it to APM systems for storage and analysis. This standardization allows applications to operate independently of specific APM APIs or data structures.
Key OpenTelemetry Packages for .NET
OpenTelemetry in .NET is implemented through several NuGet packages:
- Core API:
OpenTelemetry
: Provides core OTel functionality.
- Instrumentation:
OpenTelemetry.Instrumentation.AspNetCore
: Instrumentation for ASP.NET Core and Kestrel.OpenTelemetry.Instrumentation.GrpcNetClient
: Tracks outbound gRPC calls.OpenTelemetry.Instrumentation.Http
: Tracks outbound HTTP calls viaHttpClient
andHttpWebRequest
.OpenTelemetry.Instrumentation.SqlClient
: Traces database operations usingSqlClient
.
- Exporters:
OpenTelemetry.Exporter.Console
: Exports telemetry data to the console for diagnostic purposes.OpenTelemetry.Exporter.OpenTelemetryProtocol
: Exports using the OTLP protocol.OpenTelemetry.Exporter.Prometheus.AspNetCore
: Exports metrics to Prometheus via an ASP.NET Core endpoint.OpenTelemetry.Exporter.Zipkin
: Exports tracing data to Zipkin.
Implementing OpenTelemetry in a .NET Application
To integrate OpenTelemetry into your .NET application:
- Install NuGet Packages: Add the necessary OpenTelemetry packages to your project.
- Configure Services: In your application's startup configuration, set up OpenTelemetry services, specifying instrumentation and exporters.
- Run and Monitor: Execute your application and observe the emitted telemetry data using your chosen APM tools.
Why Use OpenTelemetry?
- Standardization: Provides a unified API and SDK to collect telemetry data.
- Interoperability: Works with multiple observability backends like Prometheus, Jaeger, Zipkin, Azure Monitor, and more.
- End-to-End Tracing: Tracks requests as they flow through distributed systems.
- Cost-Efficiency: Reduces the need for vendor-specific SDKs and tools.
Benefits for Developers
- Debugging: Quickly identify performance bottlenecks or failures.
- Scalability: Analyze distributed systems with ease.
- Flexibility: Switch between observability platforms without rewriting instrumentation code.
- Future-Proofing: Align with an industry-standard for observability.
By leveraging OpenTelemetry, Hubtel developers can build more resilient, performant, and observable applications which can ensure every interaction on our platform is delightful, performant, and reliable, aligning with our mission to drive Africa forward.
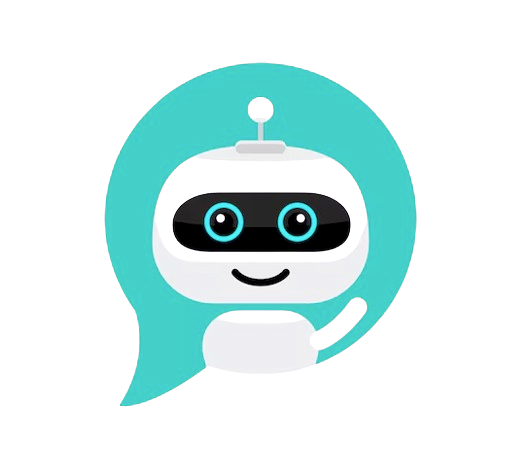
CHAT SAMMIAT