Z90 Device API
The Z90 Device API comprises implementations of PosDevice, POSPrinter and POSDeviceProcessor for the Z90 POS devices.
The API depends on classes and functionalities provided by SmartPos_1.5.6_R220425.jar, thus, this file has to be included in the libs directory of the z90 module.
Note The build.gradle.kts file of the module should be modified to load this jar file. This can be done as follows:
dependencies {
implementation(fileTree(mapOf("dir" to "libs", "include" to listOf("*.jar", "*.aar"))))
}
POSDevice Implementation for Z90
Z90Device Class
The Z90Device
class represents a specific implementation of the PosDevice
interface tailored for Z90 POS devices. It encapsulates the functionality required to interact with the Z90 device, including firmware updates, card processing, and printing capabilities.
Properties
- cardProcessor (
POSDeviceProcessor
): An instance ofZ90Processor
, which handles the processing of payment cards through the Z90 device. - cardPrinter (
POSPrinter
): An instance ofZ90Printer
, responsible for printing receipts or other documents on the Z90 device. - serialNumber (
String
): The serial number of the Z90 device, retrieved viaSystemInfoUtils.getSn()
. - deviceType (
PosDeviceType
): The type of POS device, which is set toPosDeviceType.Z90
.
Initialization
During initialization (init
block), the Z90Device
class performs a firmware update check and update if necessary by calling Z90Utils.updateFirmware(context, system)
.
Dependencies
- Context: The Android
Context
object is passed to the constructor and used within the class to perform operations such as updating firmware. - DriverManager: The
DriverManager
singleton provides access to the base system device (system
). - SystemInfoUtils: Utility class for retrieving system information, such as the serial number of the device.
- Z90Utils: Utility class containing methods for updating the firmware of the Z90 device.
This class is part of the Z90 Device API and is designed to work with the Z90 POS devices, ensuring compatibility and proper operation within the POS system.
Z90Utils Object
The Z90Utils object provides utility functions for the Z90 POS device, including firmware update, beeper control, EMV data retrieval, and SDK initialization.
Functions
getEmvData(emvHandler: EmvHandler)
: Retrieves EMV data from the given EmvHandler.beep(beeper: Beeper, freq: Int, time: Int)
: Controls the beeper with the specified frequency and duration. getFirmwareVersion(system: Sys): Retrieves the firmware version of the Z90 device.initZ90Device(context: Context, system: Sys)
: Initializes the Z90 device by updating the firmware. updateFirmware(context: Context, system: Sys): Updates the firmware of the Z90 device.initSdk(system: Sys)
: Initializes the SDK for the Z90 device.getCardHolderNameFromTrack(track: String?)
: Extracts the cardholder name from the track data.APDU_SEND_IC, APDU_SEND_RF, APDU_SEND_FELICA
: APDU commands used for processing RF cards.tags
: An array of EMV tags used for processing EMV transactions.aids
: An array of AIDs (Application Identifier) used for processing EMV transactions.
AidsAndCapks Object
The AidsAndCapks
object within Z90Utils provides functions to load various EMV applications and capks (Card Application Protocol Key) for different card networks.
loadMasterCardCapks(emvHandle: EmvHandler)
: Loads MasterCard capks into the given EmvHandler.loadVisaAIDs(emvHandle: EmvHandler)
: Loads Visa AIDs into the given EmvHandler.loadAids(emvHandler: EmvHandler)
: Loads a set of AIDs into the given EmvHandler.loadAmexCapk(ep: EmvHandler)
: Loads American Express capks into the given EmvHandler.
This documentation provides an overview of the Z90Device class and the Z90Utils object, including their properties, initialization, dependencies, and the utility functions they provide for interacting with the Z90 POS device.
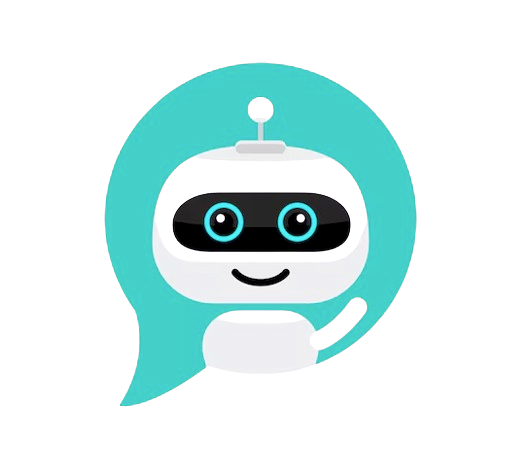
CHAT SAMMIAT