Getting Started with Aws Secrets Manager SDK
AWS Secrets Manager is a secrets management service that helps you protect access to your applications, services, and IT resources. This service enables you to easily rotate, manage, and retrieve database credentials, API keys, and other secrets throughout their lifecycle. AWS Secrets Manager SDK is a set of tools provided by Amazon Web Services (AWS) that allows developers to interact with the AWS Secrets Manager service programmatically.
Using the AWS Secrets Manager SDK, you can integrate this service into your application, scripts, or other code. This could be useful, for example, if you have an application that needs to access a database, and you want to store the database credentials securely.
The SDK provides functions to store, retrieve, and manage secrets, as well as to configure automatic secret rotation. This means you can write code that interacts with the AWS Secrets Manager service, without having to handle the underlying HTTP requests and responses yourself.
In order to get started with Hubtel.AwsSecretsManager.Sdk, do the following activities
Install-Package Hubtel.AwsSecretsManager.Sdk
Install-Package Hubtel.AwsSecretsManager.Sdk
This command is run in the Package Manager Console in Visual Studio, and it adds the SDK to your project.
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelAwsSecretsManagerSdk(c => Configuration.GetSection(nameof(AwsSecretsManagerConfig)).Bind(c));
This line of code is using the "AddHubtelAwsSecretsManagerSdk method" to add the AWS Secrets Manager SDK to the services collection, which is a way of registering dependencies in .NET. The configuration for the SDK is being pulled from the "AwsSecretsManagerConfig" section of the application's configuration
Add to appsettings.json
"AwsSecretsManagerConfig": {
"UseKeyFromConfig": true,
"AccessKeyId": "<AccessKeyId>",
"SecretAccessKey": "<SecretAccessKey>"
}
This configuration includes the AWS access key ID and secret access key, which are used to authenticate your application with AWS. The UseKeyFromConfig setting determines whether the SDK should use these keys from the configuration file.
Inject and consume service methods
[ApiController]
[Route("[controller]/[action]")]
public class AwsSecretsManagerController : ControllerBase
{
private readonly IAwsSecretsService _awsSecrets;
public AwsSecretsManagerController(IAwsSecretsService awsSecrets)
{
_awsSecrets = awsSecrets;
}
[HttpGet("{secretName}")]
public async Task<IActionResult> GetSecret(string secretName)
{
var secret = await _awsSecrets.GetSecretAsync(secretName);
return Ok(secret);
}
}
The above code defines a class AwsSecretsManagerController which inherits from ControllerBase, making it a controller in an ASP.NET Core application.
The ApiController attribute indicates that this class is a controller that responds to web API requests.
The Route attribute defines the route template for this controller. The controller placeholder will be replaced by the controller's name, and the action placeholder will be replaced by the name of the action method that handles the request.
The class has a private read-only field _awsSecrets of type IAwsSecretsService. This field is initialized through the constructor of the class, which accepts an IAwsSecretsService instance. This is an example of dependency injection.
The GetSecret method is an action method that handles HTTP GET requests. The HttpGet attribute specifies that this method should be invoked for GET requests. The secretName in the attribute is a route parameter that will be replaced by the actual value from the request URL.The method is asynchronous, denoted by the async keyword, and it returns a Task,ActionResult . This means it's a non-blocking method and returns a task representing the asynchronous operation.
Inside the GetSecret method, it calls the GetSecretAsync method of the awsSecrets service, passing the secretName as a parameter. This retrieves the secret value associated with the given secretName.
The method then returns the secret in the HTTP response using the Ok method, which creates an OkResult (HTTP 200) with the specified content.
Want to dive deeper into this SDK?See the AWS Official Documentation .
Next Topic : Hubtel AI GptDB PG SDK
Was this helpful? 📚
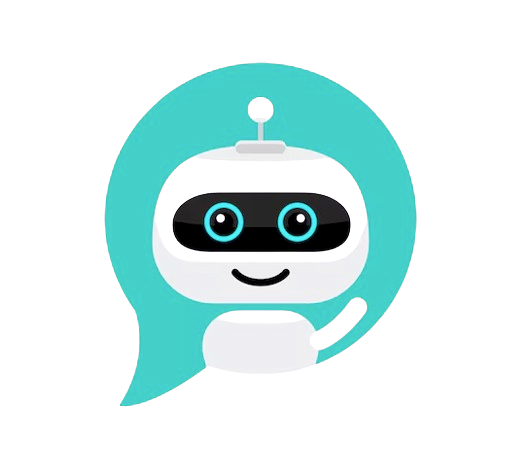
CHAT SAMMIAT