Getting Started with Hubtel Semantic Kernel Sdk
This SDK allows you to interact with lLms using Microsoft Semantic Kernel library.
In order to get started with Hubtel Semantic Kernel Sdk, do the following activities
Install-Package Hubtel.SemanticKernel.Sdk
Install-Package Hubtel.SemanticKernel.Sdk
is a command for the NuGet Package Manager Console in Visual Studio. This command installs the Hubtel.SemanticKernel.Sdk
package into the current project.
Install-Package Hubtel.SemanticKernel.Sdk
Add to appsettings.json
The
OpenAIModelName
property is set to"gpt-3.5-turbo-0301"
. This suggests that the SemanticKernel component uses the GPT-3.5 Turbo model provided by OpenAI. GPT-3.5 Turbo is a powerful language model developed by OpenAI that can generate human-like text based on the input it's given.The
OpenAIAPIKey
property is set to"{Your_API_Key}"
. This is a placeholder for the API key you would need to interact with OpenAI's API. You would replace{Your_API_Key}
with your actual OpenAI API key. This key is used to authenticate your application's requests to the OpenAI API.In summary, this configuration is used to set up and authenticate the connection between the SemanticKernel component and the OpenAI API.
"SemanticKernel": {
"OpenAIModelName" : "gpt-3.5-turbo-0301",
"OpenAIAPIKey" : "{Your_API_Key}"
}
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelSemanticKernelSdk(Configuration)
is a method call toAddHubtelSemanticKernelSdk
on theservices
object. Theservices
object is of typeIServiceCollection
, which is a framework-provided interface that is used to register services to the application's dependency injection container during the startup configuration phase.The
AddHubtelSemanticKernelSdk
method is likely an extension method that registers the Hubtel Semantic Kernel SDK services into the application's dependency injection container. This allows the SDK's services to be injected into other parts of the application where they are needed.The
Configuration
parameter being passed to the method is likely an instance ofIConfiguration
, another framework-provided interface that represents the application's configuration properties. This object is typically used to access configuration settings from sources like environment variables, command line arguments, or configuration files likeappsettings.json
. In this context,the AddHubtelSemanticKernelSdk
method uses the Configuration object to access settings specific to the Hubtel Semantic Kernel SDK.
services.AddHubtelSemanticKernelSdk(Configuration);
Inject and consume service methods
This is a C# class named
AIController
, which is a part of an ASP.NET Core application. This class is decorated with the[ApiController]
and[Route("[controller]")]
attributes, indicating that it's a controller that handles HTTP requests in a RESTful API.The
AIController
class has a private readonly field_kernel
of typeISemanticKernel
. This field is initialized via the constructor, suggesting that theISemanticKernel
implementation is injected into this controller using dependency injection.In the constructor, the
InitializeKernelBuilder
method of_kernel
is called with a large string argument. This string appears to be instructions for an AI model, specifying its behavior and the context it should use when processing input.The
AIController
class has a single public method,GetSummary
, which is an asynchronous method that returns aTask<IActionResult>
. This method is decorated with the[HttpGet("GetSummary/{query}")]
attribute, indicating that it handles HTTP GET requests at the path/AI/GetSummary/{query}
, whereAI
is the controller name inferred from the[controller]
placeholder in the class-level route attribute, and{query}
is a route parameter.Inside the
GetSummary
method, thePrompt
method of_kernel
is called with thequery
parameter, and the result is awaited. ThePrompt
method likely sends the query to the AI model and returns its response. The response is then wrapped in anOk
result (HTTP 200 OK), which is a common way to return successful responses in ASP.NET Core APIs.
[ApiController]
[Route("[controller]")]
public class AIController : ControllerBase
{
private readonly ISemanticKernel _kernel;
public AIController(ISemanticKernel kernel)
{
_kernel = kernel;
_kernel.InitializeKernelBuilder(@"You are an expert in English Grammar.
I will give you some words to correct the spelling or the sentence based on a particular context.
Do not explain anything. Just give me the correct version of the word as close to english as possible.
If you cannot find any suitable match just output 'NO_MATCH'
The context you are to use is an ecommerce inventory for groceries.
The output should be a json array of strings.
{{$input}}");
}
[HttpGet("GetSummary/{query}")]
public async Task<IActionResult> GetSummary(string query)
{
var response = await _kernel.Prompt(query);
return Ok(response);
}
}
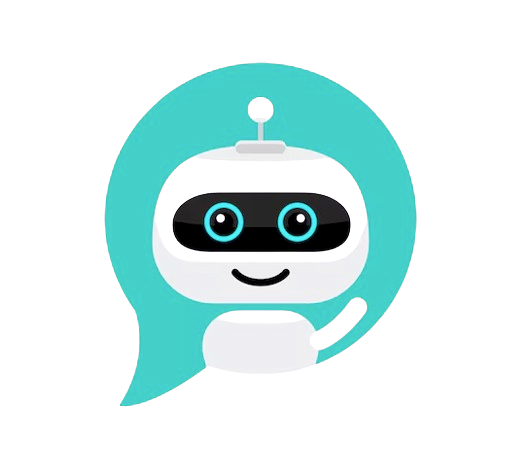
CHAT SAMMIAT