GHQR Parser
A "GHQR Parser" an SDK for parsing GHQR Codes and is tool that can read these GHQR codes and extract the information stored in them. This involves recognizing the specific format of the GHQR code, interpreting the encoded data, and converting it into a format that can be used by the application.
In essence, GHQR Parser refers to a tool or component that interprets GHQR codes, extracting and processing the information they contain.
Add the reference to your csproj, or grab it from Hubtel's Nuget Package store and update Packages.
The command below is a part of NuGet, which is a package manager for .NET. It allows developers to share and use reusable code. The Install-Package command is used to download and install Hubtel.GHQR.Sdk
package from the NuGet package repository.
Install-Package Hubtel.GHQR.Sdk
Usage
In the first line,
var qr = "--GHQR-CODE--"
, a variable namedqr
is declared and assigned a placeholder string--GHQR-CODE--
. In a real-world scenario, this would be replaced with an actual GHQR code that you want to parse.In the second line,
var result = GHQRParser.ParseGHQR(qr)
, theParseGHQR
method of theGHQRParser
class is called with theqr
variable as an argument. This method is presumably designed to parse the provided GHQR code and extract the information it contains.The result of the
ParseGHQR
method is stored in theresult
variable. The type and structure ofresult
would depend on how theParseGHQR
method is implemented, but it would typically contain the data extracted from the GHQR code in a structured format that can be easily used in the application.
var qr = "--GHQR-CODE--";
var result = GHQRParser.ParseGHQR(qr);
Result Interpretation
The GHQR code contains a lot of information. But for wha Hubtel needs currently are
- Terminal Label
- Merchant Name
- Merchant City
- Country
The GHQRParseResult
class is used to store the result of a GHQR code parsing operation. It has four properties:
Error
: a boolean indicating whether an error occurred during parsing.Exception
: if an error occurred, this property will hold the exception that was thrown.ErrorMessage
: a string that provides more information about the error.GHQRDecodedData
: an instance of theGHQRData
class that holds the parsed data from the GHQR code.
The GHQRData
class represents the data extracted from a GHQR code. It has several properties, each of which is a GhqrNode
object:
GHQRCode
: the original GHQR code.TerminalLabel
,CRC
,MerchantName
,CountryCode
,MerchantCity
: these properties represent different parts of the GHQR code.
The GhqrNode
class represents a node in the parsed GHQR data. It has several properties:
Name
andCode
: these are required properties, as indicated by the[Required]
attribute.Length
: the length of the node's value.Value
: the node's value.Children
: a list of child nodes. This allows the GHQR data to be represented as a tree structure.ChildrenMap
: a dictionary that maps string keys toGhqrNode
values. This provides a way to quickly look up child nodes by name.
The object returned from the parser is defined as
public class GHQRParseResult
{
public bool Error { get; set; }
public Exception Exception { get; set; }
public string ErrorMessage { get; set; }
public GHQRData GHQRDecodedData;
}
public class GHQRData
{
public string GHQRCode { get; set; }
public GhqrNode TerminalLabel { get; set; }
public GhqrNode CRC { get; set; }
public GhqrNode MerchantName { get; set; }
public GhqrNode CountryCode { get; set; }
public GhqrNode MerchantCity { get; set; }
}
public class GhqrNode
{
[Required] public string Name { get; set; }
[Required] public string Code { get; set; }
public string Length { get; set; }
public string Value { get; set; }
public IEnumerable<GhqrNode> Children { get; set; } = new List<GhqrNode>();
public IDictionary<string, GhqrNode> ChildrenMap { get; set; } = new Dictionary<string, GhqrNode>();
}
The GHQRData contains all the fields we need to currently proceed with a transaction. It has 6 properties, one which is the qrCode that was submitted and the remaining are GhqrNode Objects. The node contains the information decoded from the ghqrCode.
An Example
Lets say we scaned a qrCode of value
var qr = "000201010211021640458700050864210416532631101019579732790018GH.NET.GHIPSS.GHQR01166225001324402213020601000003030010416E21201223T1200005204625353039365802GH5919ISTER MEDICAL SERVI6005ACCRA62240308244022130708244022136304A474"
What we do next is to pass this qr-code to our parser
var result = GHQRParser.ParseGHQR(qr);
Result is an object of type GHQRParseResult
/*
if result.Error == true, then an error occured
All information regarding the error are passed to the calling code through the ErrorMessage field and Exception fields of GHQRParseResult
*/
var ghqrData = result.GHQRDecodedData;
//We can then retrieve our fields of interest as follows
var terminalId = ghqrData.TerminalLabel.Value;
var merchantName = ghqrData.MerchantName.Value;
var merchantCity = ghqrData.MerchantCity.Value;
var countryCode = ghqrData.CountryCode.Value;
Was this page helpful?
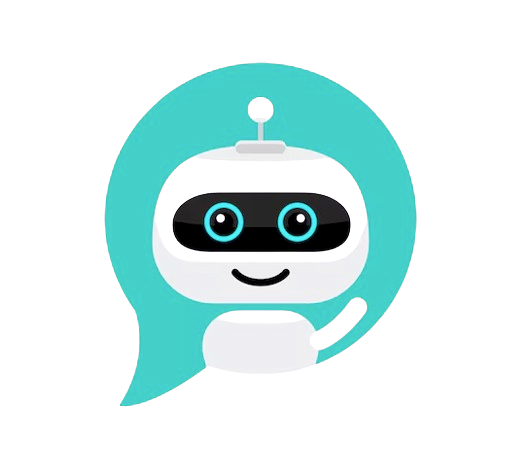
CHAT SAMMIAT