Overview
The face-verification package provides a simple interface to integrate face verification functionality into a frontend application. It offers a straightforward API to incorporate facial recognition and verification without dealing with complex implementation details.
Installation
- Copy the snippet below and paste into the .npmrc file in your project's root folder. If your project doesn't have a .npmrc file, create one.
@hubtel:registry=https://pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/
always-auth=true
; begin auth token
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:username=hubtel
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:_password=bXhsYnV1amJxeGs0YnJtaGZscjJneG55ZGx1cHdrenJpYXFxdXVhejM3eXkybm1mNXp6YQ==
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:email=npm requires email to be set but doesn't use the value
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:username=hubtel
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:_password=bXhsYnV1amJxeGs0YnJtaGZscjJneG55ZGx1cHdrenJpYXFxdXVhejM3eXkybm1mNXp6YQ==
//pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:email=npm requires email to be set but doesn't use the value
; end auth token
- Run installation command:
npm install
- Now install the package:
npm install @hubtel/face-verification
Usage
The FaceVerification class integrates face verification functionality into your application. It supports two types of initialization:
- Container Initialization
- Modal Initialization
Modal Initialization
Modal initialization involves displaying the face verification UI in a modal (popup) window that overlays the main content of your application.
TIP
This approach is particularly useful in scenarios where you want to capture the user's full attention during the verification process without permanently altering the underlying page structure.
import React, { useEffect } from "react";
import FaceVerification, { VerificationResult } from "@hubtel/face-verification";
const fv = new FaceVerification();
const Verification = () => {
const initVerificationModal = () => {
fv.initModal(
{
verificationType: "NIA",
phoneNumber: "020XXXXXX",
ghanaCard: "GHA-XXXXXXXXX-X",
privateKey: PRIVATE_KEY,
maxFailures: 4,
},
{
onSuccess: handleSuccess,
onFailure: handleFailure,
onClose: (data) => {
console.log(data);
},
}
);
};
const handleSuccess = (data: VerificationResult) => {
console.log(data);
fv.closeModal();
};
const handleFailure = (data: VerificationResult) => {
console.log(data);
};
return <button onClick={initVerificationModal}>Verify</button>;
}
export default Verification;
Here’s a table that summarizes the parameters of the initModal
method:
Parameter | Type | Description |
---|---|---|
options | object | Configuration object for the verification process. Contains the following properties: |
verificationType | string | Specifies the type of verification: "ML" (machine learning), "NIA" (National Identification Authority) or "ML_NIA" (combination of both). |
phoneNumber | string | The user's phone number (e.g., "233XXXXXXXXX"). (used when verificationType is "NIA" or "ML_NIA" .) |
ghanaCard | string | The Ghana Card number of the user, (used when verificationType is "NIA" or "ML_NIA" .) |
privateKey | string | A private key for securely communicating with the NIA verification API during verification. (used when verificationType is "NIA" or "ML_NIA" ) |
maxFailure | number | Maximum number of failed verification attempts allowed before a failure event is posted. This property has a default value of 3. |
callbacks | object | Callback functions for handling different outcomes of the verification process: |
onSuccess | function | Triggered upon successful verification. |
onFailure | function | Triggered upon verification failure. |
onClose | function | Triggered when the close icon on the verification modal is clicked. |
Container Initialization
To use the FaceVerification class and initialize the face verification UI within a specific container element on your page, you need to create a container element in your HTML with a unique ID that will serve as the container for the face verification UI.
TIP
Using a container for the face verification UI is suitable in various scenarios where you want the face verification component to be embedded within a specific part of your application’s user interface.
import React, { useEffect } from "react";
import FaceVerification from "@hubtel/face-verification";
const fv = new FaceVerification();
const Verification = () => {
useEffect(() => {
// pass element ID as argument to init function
fv.init("fv-container", {
verificationType: "NIA",
phoneNumber: "020XXXXXX",
ghanaCard: "GHA-XXXXXXXXX-X",
privateKey: PRIVATE_KEY,
maxFailures: 4,
},
{
onSuccess: handleSuccess,
onFailure: handleFailure
});
}, []);
const handleSuccess = (data: VerificationResult) => {
console.log(data);
};
const handleFailure = (data: VerificationResult) => {
console.log(data);
};
// element with ID
return <div id="fv-container"></div>;
};
export default Verification;
Here’s a table that summarizes the parameters of the initModal
method:
Parameter | Type | Description |
---|---|---|
container | string | The identifier (e.g., ID) of the HTML container element where the face verification interface will be rendered. |
options | object | Configuration object for the verification process. Contains the following properties: |
verificationType | string | Specifies the type of verification: "ML" (machine learning), "NIA" (National Identification Authority) or "ML_NIA" (combination of both). |
phoneNumber | string | The user's phone number (e.g., "233XXXXXXXXX"). (used when verificationType is "NIA" or "ML_NIA" .) |
ghanaCard | string | The Ghana Card number of the user, (used when verificationType is "NIA" or "ML_NIA" .) |
privateKey | string | A private key for securely communicating with the NIA verification API during verification. (used when verificationType is "NIA" or "ML_NIA" ) |
maxFailure | number | Maximum number of failed verification attempts allowed before a failure event is posted. This property has a default value of 3. |
callbacks | object | Callback functions for handling different outcomes of the verification process: |
onSuccess | function | Triggered upon successful verification. |
onFailure | function | Triggered upon verification failure. |
Success Callback Response
When the face verification process succeeds, the following response will be returned in the success callback:
{
success: true,
message: "Image passed all checks",
imageFile: File // file object of captured image
eventName: "success"
}
Failure Callback Response
When the face verification process fails, the following response will be returned in the failure callback:
{
"success": false,
"message": "Error Message",
"imageFile": null,
"eventType": "failure"
}
Image Validation Criteria
To ensure an image is validated successfully, it must meet the following criteria:
- Proper Lighting: The image should have proper brightness with minimal shadows.
- Plain Background: A plain, light-colored background is required.
- Resolution: The image should have a minimum resolution of 100x100 pixels.
- No Glasses: The user should not be wearing glasses.
- Centered Face: The face should be centered, fully visible, and looking straight at the camera.
- Eyes Open: Both eyes should be open and clearly visible.
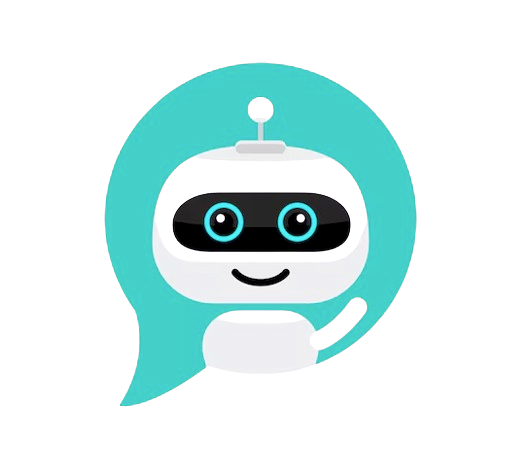
CHAT SAMMIAT