Getting Started with Hubtel AI GptDB PG SDK
This SDK allows you to bring an interactive experience to your Postgres Database. The SDK includes tools for working with artificial intelligence, related to the Generative Pretrained Transformer (GPT) models that the database is named after. The "PG" indicates that this SDK is specifically for a GPT-DB that uses PostgreSQL.
In order to get started with Hubtel.Ai.GptDb.Pg.Sdk, do the following activities
Install-Package Hubtel.Ai.GptDb.Pg.Sdk
The code snippet below is a command that's used in the NuGet Package Manager Console in Visual Studio. This command is written in PowerShell and installs the Hubtel.Ai.GptDb.Pg.Sdk
into the current .NET project.
Install-Package Hubtel.Ai.GptDb.Pg.Sdk
Add to appsettings.json
The
ConnectionString property
is set to"{YOUR_PG_CONN_STRING}"
. This is a placeholder for the connection string to a PostgreSQL database. You would replace{YOUR_PG_CONN_STRING}
with your actual PostgreSQL connection string. This connection string is used to connect to the PostgreSQL database that underlies the GPT-DB (Generative Pretrained Transformer Database) in your application.The
OpenAiApiKey
property is set to"{YOUR_OPEN_AI_KEY}"
. This is a placeholder for the API key you would need to interact with OpenAI's API. You would replace{YOUR_OPEN_AI_KEY}
with your actual OpenAI API key. This key is used to authenticate your application's requests to the OpenAI API.This configuration is used to set up the connection to the PostgreSQL database and authenticate the connection to the OpenAI API for the GptDbConfig component in your application.
"GptDbConfig":{
"ConnectionString": "{YOUR_PG_CONN_STRING}",
"OpenAiApiKey":"{YOUR_OPEN_AI_KEY}"
}
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelGptDbPg(c => _configuration.GetSection(nameof(GptDbConfig)).Bind(c))
is a method call toAddHubtelGptDbPg
on theservices
object. The services object is of typeIServiceCollection
, which is a framework-provided interface that is used to register services to the application's dependency injection container during the startup configuration phase.The
AddHubtelGptDbPg
method is likely an extension method that registers the Hubtel GPT-DB PostgreSQL SDK services into the application's dependency injection container. This allows the SDK's services to be injected into other parts of the application where they are needed.The method takes
c => _configuration.GetSection(nameof(GptDbConfig)).Bind(c)
as an argument. This lambda expression is used to configure the options for the Hubtel GPT-DB PostgreSQL SDK. It retrieves theGptDbConfig
section from the application's configuration (represented by_configuration
), and binds it to the options object (c
). This means that the settings in the GptDbConfig section of the configuration will be used to configure the SDK.
services.AddHubtelGptDbPg(c => _configuration.GetSection(nameof(GptDbConfig)).Bind(c));
Inject and consume service methods
The
[ApiController]
attribute is used to mark this class as a controller that responds to web API requests. The[Route("[controller]")]
attribute sets the route template for the controller, where[controller]
is a placeholder for the controller's name.The
[ProducesResponseType(StatusCodes.Status500InternalServerError, Type = typeof(ApiResponse<EmptyDto>))]
attribute indicates that actions in this controller can return a status code of 500 (Internal Server Error), with a response body of typeApiResponse<EmptyDto>
. The[Authorize(AuthenticationSchemes = "Bearer")]
attribute specifies that the controller requires Bearer token authentication.The
GptDbController
class has two private readonly fields,_logger
and_gptDbPgEngine
, which are initialized via the constructor._logger
is of typeILogger<GptDbController>
, which is used for logging._gptDbPgEngine
is of typeIGptDbPgEngine
, which is an interface defining operations related to the GPT-DB PostgreSQL engine.The class has two action methods,
GptPg
andGptPgExamples
. Both methods are decorated with several attributes that define their behavior as action methods, such as HTTP method([HttpPost]
or[HttpGet])
, route ([Route("pg")]
or[Route("pg/examples")]
), and response type ([ProducesResponseType(StatusCodes.Status200OK)]
). Both methods are asynchronous, returning aTask<IActionResult>
.The
GptPg
method takes two parameters,question
andisSqlOnly
, and uses the_gptDbPgEngine
to generate SQL or query the database based on thequestion
andisSqlOnly
parameters.The
GptPgExamples
method takes acount
parameter and uses the_gptDbPgEngine
to fetch a specified number of query samples.
[ApiController]
[Route("[controller]")]
[ProducesResponseType(StatusCodes.Status500InternalServerError, Type = typeof(ApiResponse<EmptyDto>))]
[Authorize(AuthenticationSchemes = "Bearer")]
public class GptDbController : HubtelApiControllerBase
{
private readonly ILogger<GptDbController> _logger;
private readonly IGptDbPgEngine _gptDbPgEngine;
public GptDbController(ILogger<GptDbController> logger
,IGptDbPgEngine gptDbPgEngine)
{
_logger = logger;
_gptDbPgEngine = gptDbPgEngine;
}
/// <summary>
/// Translates natural language to PG SQL
/// </summary>
/// <param name="question"></param>
/// <param name="isSqlOnly"></param>
/// <returns></returns>
[HttpPost]
[AllowAnonymous]
[Route("pg")]
[Consumes(MediaTypeNames.Application.Json)]
[Produces(MediaTypeNames.Application.Json)]
[ProducesResponseType(StatusCodes.Status200OK)]
[SwaggerOperation(nameof(GptPg), OperationId = nameof(GptPg))]
public async Task<IActionResult> GptPg(string question,bool isSqlOnly = false
)
{
PgDbQueryResult response = null;
if (isSqlOnly)
{
response = await _gptDbPgEngine.GenerateSqlOnly(question);
}
else
{
response = await _gptDbPgEngine.QueryDb(question);
}
return Ok(response);
}
/// <summary>
/// lists examples of PG SQL queries in natural language
/// </summary>
/// <param name="count"></param>
/// <returns></returns>
[HttpGet]
[AllowAnonymous]
[Route("pg/examples")]
[Consumes(MediaTypeNames.Application.Json)]
[Produces(MediaTypeNames.Application.Json)]
[ProducesResponseType(StatusCodes.Status200OK)]
[SwaggerOperation(nameof(GptPgExamples), OperationId = nameof(GptPgExamples))]
public async Task<IActionResult> GptPgExamples(int count = 10)
{
var response = await _gptDbPgEngine.FetchQuerySamples(count);
return Ok(response);
}
}
Was this page helpful?
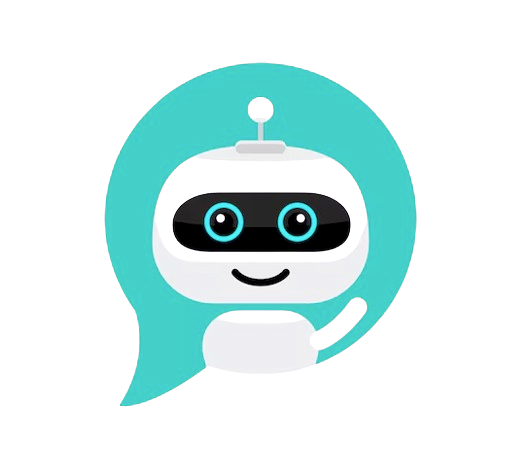
CHAT SAMMIAT