Skip to content
Getting Started with Fluent Validation
Fluent Validation is a .NET library which provides a simple and fluent way to define validation rules in your code.
In order to get started with Fluent Validation, do the following activities
Installing FluentValidation Package
Install-Package FluentValidation
Configuration
Create Validator class and define validation rules
After installing the FluentValidation package, create validator classes that define validation rules for your models
c#
public class PersonValidator : AbstractValidator<Person>//validation rules for Person model/class
{
public PersonValidator()
{
RuleFor(p => p.FirstName).NotEmpty().WithMessage("First Name is required.");
RuleFor(p => p.Age).InclusiveBetween(18, 99).WithMessage("Age must be between 18 and 99.");
// Add more validation rules as needed
}
}
Configure Fluent Validation in Startup or configuration class
c#
services.AddValidatorsFromAssemblyContaining<PersonValidator>();
services.AddMvc()
.AddFluentValidation(fv => fv.RegisterValidatorsFromAssemblyContaining<PersonValidator>());
Sample Usage
After installing and configuring FluentValidation, this is a sample use case to see how it works.
Class with properties to validate
c#
public class Person
{
public string FirstName { get; set; }
public int Age { get; set; }
}
Inject Validator interface into Controllers and Validate data
c#
public class PersonController : Controller
{
private readonly IValidator<Person> _personValidator;
public PersonController(IValidator<Person> personValidator)
{
_personValidator = personValidator;
}
[HttpPost]
public IActionResult CreatePerson(Person person)
{
var validationResult = _personValidator.Validate(person);
if (!validationResult.IsValid)
{
return BadRequest(validationResult.Errors);
}
return Ok();
}
}
Further Readings
Follow this link to learn more.
Was this helpful? 📚
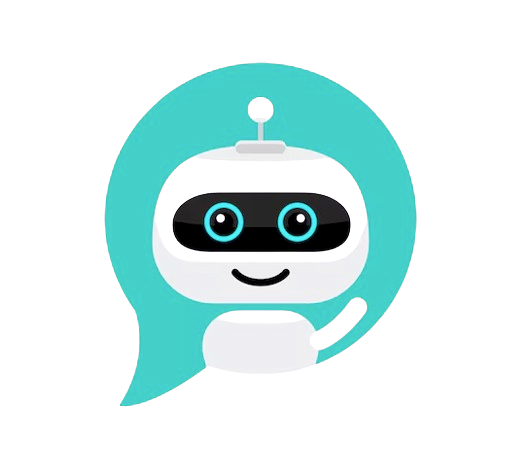
CHAT SAMMIAT