Tutorial & Usage - Core Network
Overview
This section tutors developers on how to configure and use the core network library in their mobile applications. In this tutorial, we are going to use core network in our sample app called Hubsell to fetch user details.
Installation
The core network library is situated in core platform hence to use it, developers must add core platform to their project.
dependencies:
core_platform:
git:
url: [email protected]:v3/hubtel/Mobile-Apps/Platform-Library-Flutter-Core-Platform
ref: 1.0.36 //Use updated version
Setting Up Requester
To begin making requests with the core network library, it is a requirement to have a requester class that extends or implements the IAPIRequester abstract class. Implementing/Extending the IApiRequester abstract class gives you access to the makeRequest
method to make requests.
Example:
class HubsellRequesterClass extends IApiRequester{
@override
IAnalyticsUtils analyticsUtils = HubsellAnalyticsUtils.instance;
@override
Serializable? defaultEventData;
RequesterClass({this.defaultEventData});
@override
Future<Map<String, String>> getDefaultHeaders() {
return Future.value({
"Content-Type": "application/json",
"Accept": "application/json",
});
}
}
Setting Up An Api Core Class
An api core class should be a class in your project, that extends ApiCore with ApiEndPointCore as Mixin. Extending ApiCore gives you access to the requester property hence has be initialized in a constructor and ApiEndpoint core gives you access to the createEndPoint
method to create an APIEndPoint
object.
class HubsellApi extends ApiCore with ApiEndPointCore{
HubsellApi({required super.requester});
}
Adding Requester Methods.
In our HubsellApi
, we will now add methods to help us make requests.
class HubsellApi extends ApiCore with ApiEndPointCore{
HubsellApi({required super.requester});
Future<ApiResponse<DataResponse<User>>> getUserDetails(
{required Serializable request}) async {
final result = await requester.makeRequest(
apiEndPoint: createEndpoint(
requestType: HttpVerb.POST,
headers: _defaultHeaders,
authority: 'hubsell.com',
path: '/api/getUserInfo',
body: request.toMap(),
),
);
final data = DataResponse<User>.fromJson(
result.response,
(x) => User.fromJson(x),
);
return ApiResponse(response: data, status: result.status);
}
}
Using Our Api Requester and Api Core class.
In our sample apps we would like our view model to make requests on behalf of our views.
Creating The View Model.
class HubsellViewModel {
final requester = HubsellRequesterClass(defaultEventData: {"name":"Hubsell"})
late HubsellApi api = HubsellApi(requester: requester);
Future<ApiResponse<DataResponse<User>>> fetchUserDetailsFromApi(
{required Serializable request}) async {
final userDetails = await api.getUserDetails(request: UserInfo(phone: "233556236701"));
return userDetails;
}
}
Conclusion
In this tutorial, we have covered the essential steps for configuring and using the core network library within a mobile application. By setting up a requester class and an API core class, we enabled our sample app, Hubsell, to make structured API requests efficiently. We demonstrated creating a Requester class to handle request details, a core API class to manage endpoints, and a view model to handle interactions between the view and the API.
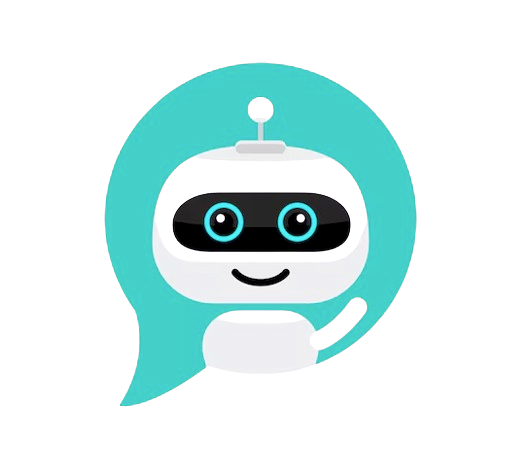
CHAT SAMMIAT