Modal Integration
The modal integration method opens the checkout page in a modal popup. This is done by calling the openModal
method of the Checkout SDK instance and passing the purchase information, configuration options and callBacks for event handling as parameters.
Installation
To use the Hubtel Checkout SDK to collect payment, install the NPM Package or include the the CDN link in your HTML file:
npm install @hubteljs/checkout
Then import it in the component where you want to use it
import CheckoutSdk from "@hubteljs/checkout";
TIP
Note: Before calling the Checkout SDK, most internal apps would call a Pre-Checkout API provided by their backend team.
Sample Pre-checkout Request:
{
"amount": "1",
"phoneNumber": "0200000000"
}
Sample Pre-checkout Response:
{
"message": "success",
"code": "200",
"data": {
"description": "Payment of GHS 1.00 for (18013782) (MR SOMUAH STA ADANE-233557913587) ",
"clientReference": "f03dbdcf8d4040dd88d0a82794b0229f",
"amount": 1.0,
"customerMobileNumber": "233557913587",
"callbackUrl": "https://instantservicesproxy.hubtel.com/v2.1/checkout/callback"
}
}
The response from the Pre-Checkout API usually contains all information needed for a successful checkout. Once retrieved the data is then passed onto the Hubtel Checkout SDK to begin the checkout.
:::
Example Usage
Collect the purchase information, configuration options and callbacks and pass them to openModal
the method of the Checkout SDK instance.
<!-- In your HTML -->
<form id="checkout-form">
<div>
<label for="amount">Amount:</label>
<input type="number" id="amount" name="amount" required />
</div>
<div>
<label for="description">Description:</label>
<input type="text" id="description" name="description" required />
</div>
<div>
<label for="phone">Customer Phone Number:</label>
<input type="tel" id="phone" name="phone" required />
</div>
<button type="submit">Pay Now</button>
</form>
<div id="checkout-iframe"></div>
// In your javascript code
document
.getElementById("checkout-form")
.addEventListener("submit", function (event) {
event.preventDefault();
const amount = document.getElementById("amount").value;
const description = document.getElementById("description").value;
const phone = document.getElementById("phone").value;
const checkout = new CheckoutSdk();
const purchaseInfo = {
amount: amount,
purchaseDescription: description,
customerPhoneNumber: phone,
clientReference: "unique-client-reference-12345",
};
const config = {
branding: "enabled",
callbackUrl: "https://yourcallbackurl.com", // Replace with your callback URL
bearerToken: "[TOKEN]", // Replace with your actual token.
integrationType: "Internal",
branchId: "7", // Replace with branchId
businessId: "ericacormey", // Replace with businessId
};
checkout.openModal({
purchaseInfo,
config,
callBacks: {
onInit: () => console.log("Iframe Initialized"),
onPaymentSuccess: (data) => console.log("Payment Success", data),
onPaymentFailure: (data) => console.log("Payment Failure", data),
onLoad: () => console.log("Iframe Loaded"),
onFeesChanged: (fees) => console.log("Fees Changed", fees),
onResize: (size) => console.log("Iframe Resized", size?.height),
},
});
});
Parameters
Parameter | Type | Description |
---|---|---|
purchaseInfo | Object | Contains details about the purchase. |
amount | number | The purchase amount. |
purchaseDescription | string | A brief description of the purchase. |
customerPhoneNumber | string | The customer’s phone number. |
clientReference | string | A unique reference for the purchase. |
config | Object | Contains configuration options. |
branding | string | Set to "enabled" to show Hubtel branding or "disabled" to hide it. |
callbackUrl | string | The URL to which the SDK will send the response. |
bearerToken | string | Bearer Token |
integrationType | string | Specifies the integration type. Must be set to "Internal", which is the type used for internal integration. |
branchId | string | Yes |
businessId | string | The ID of the business |
callacks | Object | Callback functions for various events. |
onInit | function | Called when the iframe is initialized. |
onPaymentSuccess | function | Called when the payment is successful. |
onPaymentFailure | function | Called when the payment fails. |
onLoad | function | Called when the iframe is loaded. |
onFeesChanged | function | Called when the fees change. |
onClose | function | Called when the the modal is closed. |
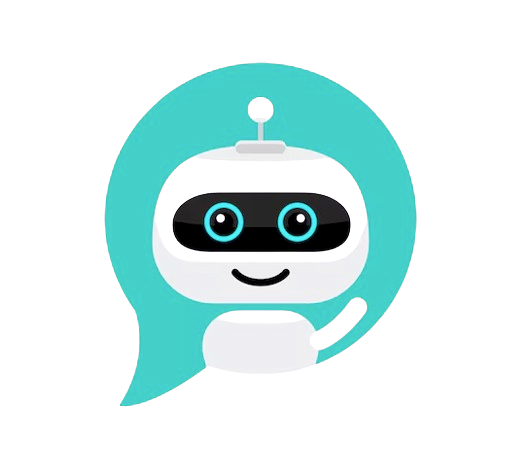
CHAT SAMMIAT