Skip to content
Logging Best Practices
To ensure effective logging and observability with the Hubtel Event Publisher, follow these best practices when implementing logging in your application:
1. Standardize Event Structures
- Ensure to follow as much as possible the structure defined for each log level.
- Include all necceaary properties such as:
eventType
: Categorize events (e.g.,Error
,Regular
,Transaction
).actionName
: Describe the specific action (e.g.,user_logged_in
,fetch_failed
).
Example:
typescript
eventPub.info({
eventType: "Transaction",
actionName: "order_placed",
additionalProperties: { orderId: "12345", amount: 99.99 },
});
2. Log Meaningful Information
- Avoid logging overly verbose or irrelevant details. Focus on actionable and useful data.
- Include enough context to understand the issue or event without requiring additional data retrieval.
Example: Log the API endpoint and response code for debugging:
typescript
eventPub.error({
eventType: "Error",
actionName: "api_request_failed",
endpoint: "/users",
statusCode: 500,
});
3. Use Appropriate Log Levels
- Match the severity of the event to the appropriate log level:
info
: Routine operations or successful actions.warn
: Non-critical issues that require attention.error
: Critical problems affecting functionality.debug
: Detailed information useful during development.
Example:
typescript
eventPub.warn({
eventType: "Security",
actionName: "unauthorized_access_attempt",
additionalInfo: { ip: "192.168.1.1" },
});
4. Avoid Logging Sensitive Data
- Do not log sensitive information like passwords, access tokens, or personal identification numbers (PINs).
- If sensitive information must be referenced, ensure it is obfuscated or masked.
Example: Mask user data:
typescript
eventPub.info({
eventType: "Security",
actionName: "user_login_attempt",
additionalInfo: { username: "johndoe", ip: "192.168.1.1" },
});
5. Limit Client-Side Logs
- Avoid overloading client-side logging with excessive events to reduce performance impact.
- Log only essential actions and errors to maintain a smooth user experience.
6. Monitor and Review Logs
- Periodically review logs to identify patterns, recurring issues, or unnecessary entries.
- Use insights to improve logging strategies and enhance application performance.
7. Avoid Log Overhead
- Be mindful of log sizes and frequency to minimize storage costs and network overhead.
Example: Combine similar logs into a single entry:
typescript
eventPub.info({
eventType: "Batch",
actionName: "bulk_user_update",
additionalInfo: { updatedUserIds: [101, 102, 103] },
});
Adhering to these best practices ensures that your logs remain actionable, efficient, and valuable for monitoring and debugging.
Was this helpful? 📚
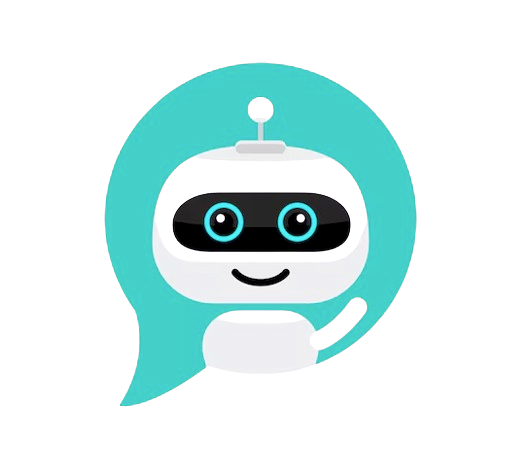
CHAT SAMMIAT