Unit Testing
This document provides guidance for engineers on unit testing practices using online training resources and adhering to the Hubtel unit testing standard. Unit testing is an essential part of software development that verifies the correctness of individual units or components of code. By following these guidelines, engineers can ensure the reliability and maintainability of their codebase.
TL;DR
This document provides guidance on unit testing practices at Hubtel. Key points include:
- Benefits: Early bug detection, improved code quality, documentation, and refactoring safety.
- Implementation: Steps to install xUnit, create test classes, and run tests.
- Best Practices: Isolate tests, keep them fast, and test edge cases.
- Setup: Install Hubtel's unit test template and add necessary dependencies.
- Execution: Steps to run unit tests in your development environment.
Benefits of Unit Testing
- Early Bug Detection: Helps catch bugs early in the development cycle.
- Code Quality: Encourages developers to write modular and maintainable code.
- Documentation: Unit tests can serve as documentation for how a function or class is supposed to behave.
- Refactoring Safety: Provides confidence when refactoring code that existing functionality remains intact.
Implementing Unit Tests in C#
To implement unit tests, we use xUnit testing framework. Below is a basic example using xUnit:
Install xUnit via NuGet:
bashdotnet add package xunit dotnet add package xunit.runner.visualstudio
Create a Test Class:
csharpusing Xunit; public class CalculatorTests { [Fact] public void Add_TwoNumbers_ReturnsSum() { // Arrange var calculator = new Calculator(); // Act int result = calculator.Add(2, 3); // Assert Assert.Equal(5, result); } }
Run the Tests: You can run the tests using the command line or a test runner integrated into Visual Studio.
Best Practices for Unit Testing
- Isolate Tests: Ensure each test is independent and does not rely on external factors.
- Keep Tests Fast: Unit tests should be quick to execute.
- Test Edge Cases: Consider various inputs, including edge cases, to ensure comprehensive coverage.
Setting Up Unit Testing
To set up unit testing in your project, follow these general steps:
- Install the Hubtel template for unit testing
dotnet new --install Hubtel.Templates.V6.UnitTests.Pack --nuget-source https://pkgs.dev.azure.com/hubtel/_packaging/hubtel/nuget/v3/index.json --interactive
- Create a new unit test project following the Hubtel standard, using the Unit Test Template
- Add the necessary dependencies or packages for the chosen unit testing framework to your project.
Running Unit Tests
To execute your unit tests, follow these steps:
- Open your project in your chosen development environment or IDE.
- Build your project to ensure that all necessary dependencies are resolved.
- Locate the unit test runner or testing window provided by your development environment.
- Run the tests using the appropriate command or button.
- Observe the test results, which should indicate whether each test passed or failed.
Summary
Unit testing is crucial for verifying the correctness of individual code units and ensuring the reliability and maintainability of the codebase. This guide outlines the following key points:
- Understand the prerequisites: Basic programming language knowledge, familiarity with Unit Testing concepts and Hubtel unit testing standard, and a development environment.
- Set up unit testing: Configure your development environment to support unit testing.
- Write effective unit tests: Follow naming conventions, organize tests logically, achieve comprehensive test coverage, use the AAA pattern (Arrange, Act, Assert), isolate tests, use readable assertions, provide relevant test data, avoid test dependencies, and keep tests fast and deterministic.
- Run unit tests: Open your project, build dependencies, locate the unit test runner, and execute the tests.
Happy unit testing!
Was this page helpful?
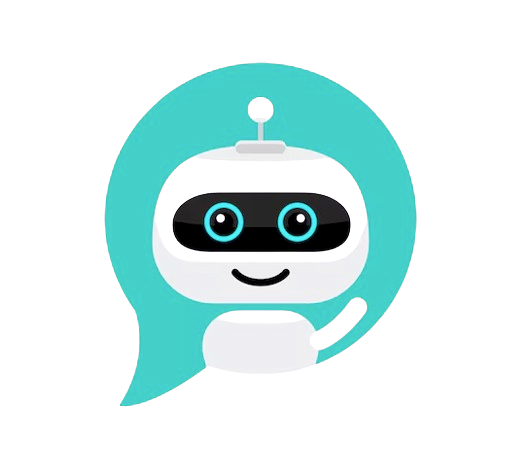
CHAT SAMMIAT