Project Structure
Our mobile architecture defines the structure and framework for developing mobile apps. It encompasses the folder structure, naming conventions, and overall design principles. This documentation facilitates seamless onboarding for developers transitioning to our team, ensuring efficient development processes and consistency across projects.
Feature
A feature constitutes a standalone product addressing specific market needs, delivering value to end-users, and serving business-facing purposes. It can be deployed independently as an app and is developed by a dedicated team. Each feature relies on the core-platform library, which provides foundational code for platform and UX functionalities, including network operations, storage management, analytics, and utilities.
Folder Structure for Features Every feature comprises two main folders:
- platform: Contains platform-specific code and functionalities.
- ux: Houses UX-related components, views, and utilities.
This structure ensures clear organization and separation of concerns within feature development, promoting maintainability and scalability.
flutter_project/
├── platform/
│ ├── datasource/
│ │ ├── persistence/
│ │ │ ├── pref_manager
│ │ │ ├── data_stores
│ │ │ └── models
│ │ └── api/
│ │ ├── api_1/
│ │ │ ├── models/
│ │ │ │ ├── api_1_request_models
│ │ │ │ └── api_1_response_models
│ │ │ ├── api_1_end_points
│ │ │ └── api_1_requests
│ │ ├── endpoints
│ │ └── requester
│ ├── analytics
│ └── utils - reusable utility and extension functions for platform
├── ux/
│ ├── navigation
│ ├── models
│ ├── resources/
│ │ ├── strings
│ │ ├── colors
│ │ ├── drawables
│ │ ├── dimens
│ │ ├── fonts
│ │ ├── regex
│ │ ├── theme
│ │ └── constants
│ ├── views/
│ │ └── feature_view_1/
│ │ ├── components/
│ │ │ └── feature_view_1_component_1.dart
│ │ ├── feature_view_screen_1.dart
│ │ └── feature_view_model.dart
│ └── shared/
│ ├── views
│ ├── view_models
│ └── utils - reusable utility and extension functions for ux
Core-Platform
The cornerstone of our mobile architecture is the core-platform library, which serves as the foundation for our app development efforts. This library comprises two essential facets:
Platform
The platform aspect of the core-platform library encompasses fundamental functionalities and utilities essential for building robust mobile applications. It includes features such as:
- API requester
- System or platform utility functions
- Storage functionalities (shared preferences, database, or file management)
UX
The UX facet of the core-platform library focuses on user experience components and resources vital for creating intuitive and visually appealing mobile interfaces. It provides:
- Views
- Components
- Navigation elements
- Color schemes
- Other essential resources (e.g., button components, empty state representations)
REST API Integration
In the Hubtel architecture, the core-platform library includes utility functions necessary for implementing REST APIs. To facilitate parsing of API responses, your class should implement the Serializable interface, which provides a toMap function for converting your class to a Map<String, dynamic>
representation.
API Response Handling
The core-platform library provides several classes to assist in parsing API responses
ApiResponse
: Parses the JSON response into status and response components. The response component is of typeT
, which extends Serializable.DataResponse
: Decodes the response from ApiResponse and provides the data component, also of typeT
extending Serializable.ListDataResponse
: Provides a list of objects where each object extendsSerializable
.PrimitiveDataResponse
: Provides responses containing primitive typesT
, whereT
does not necessarily extendSerializable
.PrimitiveListResponse
: Provides responses containing primitive typesList<T>
, whereT
does not necessarily extendSerializable
.
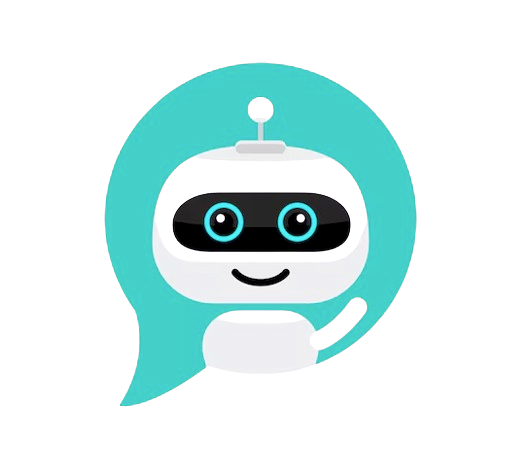
CHAT SAMMIAT