Getting Started with ES SDK
This framework helps you to interact with Elastic Search.Elasticsearch is a powerful open-source, RESTful search and analytics engine that allows you to store, search, and analyze big volumes of data in real time. It is often used as the underlying engine/technology that powers applications that have complex search features and requirements.
Installation
To install the SDK, run the following command in the Package Manager Console:
Install-Package Hubtel.ElasticSearch.Sdk
Setup in appsettings.json
The ElasticConfiguration
object contains a Hosts
array, each element of which represents a different Elasticsearch host that the application can connect to.
Each host object has several properties:
Url
: The URL of the Elasticsearch instance.Alias
: An alias for this host.BasicAuthConfig
: An object containing settings for basic authentication. IfEnableBasicAuth
istrue
, theUsername
andPassword
will be used for authentication.AwsCognitoConfig
: An object containing settings for AWS Cognito authentication. IfEnableAwsCognito
istrue
,the ServiceName
,Region
,AccessKeyId
, andAccessKeySecret
will be used for authentication.Indexes
: An array of index objects. Eachindex
object has an Index (the name of the index), anAlias
(an alias for the index), andEnableVerboseLogging
(a boolean indicating whether verbose logging is enabled for this index).This configuration allows the application to connect to one or more Elasticsearch hosts, authenticate using either basic authentication or AWS Cognito, and interact with specific indexes on each host.Place these entries in appsettings.json.Also ensure you have your ElasticConfiguration as depicted below:
"ElasticConfiguration": {
"Hosts": [
{
"Url": "<insert_url>",
"Alias": "<alias>",
"BasicAuthConfig": {
"EnableBasicAuth": false,
"Username": "<username>",
"Password": "<password>"
},
"AwsCognitoConfig": {
"EnableAwsCognito": false,
"ServiceName": "<service_name>",
"Region": "<region>",
"AccessKeyId": "<access_key_id>",
"AccessKeySecret": "access_key_secret"
},
"Indexes": [
{
"Index": "<index>",
"Alias": "<alias>",
"EnableVerboseLogging": true
}
]
}
]
}
Register the service in Startup.cs
The line
services.AddHubtelElasticSearchSdk(c => Configuration.GetSection(nameof(ElasticConfiguration)).Bind(c))
is adding the Hubtel ElasticSearch SDK to the service collection. This makes the SDK available to be injected into other parts of the application where it's needed.The
AddHubtelElasticSearchSdk
method is a custom extension method that likely sets up the necessary services for the SDK. It takes a lambda function as a parameter, which is used to configure the SDK.The
c => Configuration.GetSection(nameof(ElasticConfiguration)).Bind(c)
is retrieving theElasticConfiguration
section from the application's configuration (usually defined inappsettings.json
or another configuration source), and binding it to the SDK's configuration object. This allows the SDK to be configured based on the settings defined in theElasticConfiguration
section.In essence, this line of code is setting up the Hubtel ElasticSearch SDK with the settings defined in the
ElasticConfiguration
section of the application's configuration.
Do as shown:
services.AddHubtelElasticSearchSdk(c => Configuration.GetSection(nameof(ElasticConfiguration)).Bind(c));
Inject Service
The
OrderService
class has a private readonly field_ordersEsRepo
of typeIElasticRepository
. This field is used to interact with Elasticsearch.The constructor of the
OrderService
class takes anIElasticRepositoryFactory
as a parameter. This factory is used to create an instance ofIElasticRepository
by calling theGetElasticRepository
method with an alias and an index. The createdIElasticRepository
instance is then assigned to the_ordersEsRepo
field.The
OrderService
class has a methodGetOrder
that takes anorderId
as a parameter. This method uses the_ordersEsRepo
field to call theGetByIdAsync
method, passing theorderId
as a parameter. TheGetByIdAsync
method returns a response that contains the order data from Elasticsearch.The
GetOrder
method checks if the response is valid by accessing theIsValid
property of the response. If the response is valid, it returns theSource
property of the response, which contains the order data. If the response is not valid, it returnsnull
.Inject
IElasticRepositoryFactory
in your code to createIElasticRepository
to interact with ES.
public class OrderService
{
private readonly IElasticRepository _ordersEsRepo;
public OrderService(IElasticRepositoryFactory esRepoFactory)
{
_ordersEsRepo = esRepoFactory.GetElasticRepository("<alias>", "<index>");
}
public async Task<Order?> GetOrder(string orderId)
{
var response = await _esRepo.GetByIdAsync<Order>(orderId);
return response.IsValid ? response.Source : null;
}
}
Was this helpful? 📚
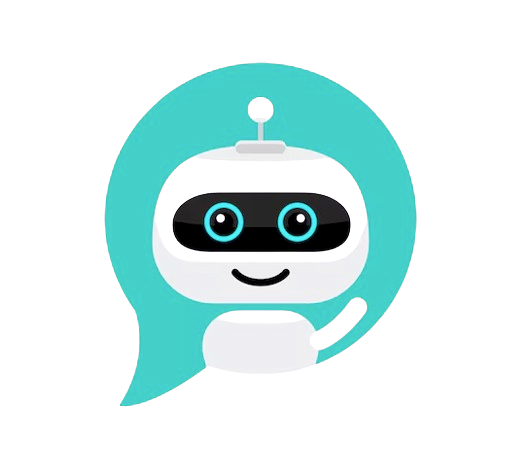
CHAT SAMMIAT