Getting Started with Inventory SDK
Inventory SDK provides tools and libraries that make it easier for developers to implement inventory management functionality in their applications. This could include features like tracking inventory levels, managing product information, handling orders and stock adjustments, and integrating with other systems like e-commerce platforms or warehouse management systems.
This SDK helps you to interact with Inveontory API.
In order to get started with Hubtel.Inventory.Sdk, do the following activities
Install-Package Hubtel.Inventory.Sdk
The provided command is a PowerShell command used in the NuGet Package Manager Console in Visual Studio to install a NuGet package.In this case, it's being used to install the Hubtel.Inventory.Sdk
package.
Install-Package Hubtel.Inventory.Sdk
Add to appsettings.json
The "InventoryConfig" block contains two properties:
"ProgrammableServiceUrl"
: This property is expected to hold the URL of a service endpoint. The placeholder text suggests that this endpoint provides some sort of programmable service details related to inventory management. In a real-world application, the placeholder would be replaced with the actual URL of the service."Timeout"
: This property specifies a timeout value in seconds. The comment indicates that the default value for this property is 45 seconds, but it has been set to 30 seconds in this case. This timeout could be used for any network requests made to the "ProgrammableServiceUrl", or for other operations related to the inventory service.
"InventoryConfig": {
"ProgrammableServiceUrl": "http://programmable service detail endpoint",
"Timeout": 30 //in seconds. Default is 45 seconds
}
Add to IServiceCollection in Program.cs or Startup.cs
services.Configure<InventoryConfig>(Configuration.GetSection(nameof(InventoryConfig)))
- This line is configuring an instance of
InventoryConfig
with the values from theInventoryConfig
section of the application's configuration (usually found inappsettings.json
). TheInventoryConfig
class would be a custom class defined in your application that matches the structure of theInventoryConfig
section in your configuration file.
services.AddHubtelInventorySdk()
- This line is adding the Hubtel Inventory SDK to the application's services. This is presumably a method provided by the
Hubtel.Inventory.Sdk
package that sets up any necessary services for the SDK to function, such as HTTP clients, database contexts, or other dependencies.
services.Configure<InventoryConfig>(Configuration.GetSection(nameof(InventoryConfig)));
services.AddHubtelInventorySdk();
Inject and consume service methods
private readonly IInventoryApi _inventoryApi
- This line declares a private, read-only field of type
IInventoryApi
named_inventoryApi
. This interface presumably provides methods for interacting with an inventory system.
var resp = await _inventoryApi.GetProgrammableServiceDetail("programmable service ID here")
This line calls the
GetProgrammableServiceDetail
method of the_inventoryApi
object, passing a placeholder string as the programmable service ID. This method is asynchronous, as indicated by theawait
keyword, so it returns a task that represents the ongoing operation. Theawait
keyword also unwraps the result of the task once it's complete. The result of the operation is stored in the resp variable.If the
HasException
property of the response istrue
, it means that an exception occurred while trying to get the programmable service detail. In this case, the code inside the firstif
block will be executed.If no exception occurred and the
IsSuccessful
property of the response istrue
, it means that the operation was successful. In this case, the code inside theelse if
block will be executed.
private readonly IInventoryApi _inventoryApi;
var resp = await _inventoryApi.GetProgrammableServiceDetail("programmable service ID here");
if(resp.HasException()){
// your logic
}
else if(resp.IsSuccessful){
//..your logic
}
Was this helpful? 📚
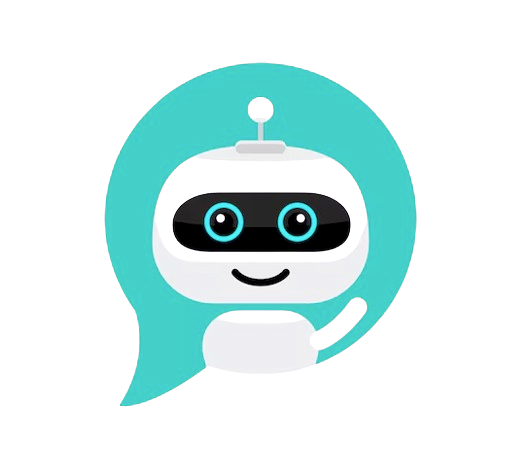
CHAT SAMMIAT