Skip to content
Integration Testing
What is Integration Testing?
Integration Testing is a phase in software testing where individual components are combined and tested as a group. This ensures that an app's components function correctly at a level that includes the app's supporting infrastructure, such as the database, file system, and network.
Benefits of Integration Testing
- Detect Interface Issues: Helps identify issues with the way components interact.
- Verify Data Flow: Ensures that data is passed correctly between modules.
- Improves System Reliability: Increases confidence that the system behaves correctly as a whole.
- Early Issues Detection: Detect problems in the interaction between modules early in the development process.
- Ensures Compatibility: Confirm that different components work together seamlessly.
- Prevents Regressions: Catch issues that could arise from changes in integrated components.
Implementing Integration Tests in C#
Integration tests in C# often involve testing multiple components together, such as services, databases, and APIs. Here's an example:
Create a Test Class:
csharpusing Xunit; public class OrderServiceIntegrationTests { [Fact] public void PlaceOrder_ValidOrder_StoresInDatabase() { // Arrange var orderService = new OrderService(new OrderRepository()); // Act orderService.PlaceOrder(new Order { Id = 1, ProductName = "Laptop", Quantity = 1 }); // Assert var savedOrder = orderService.GetOrder(1); Assert.Equal("Laptop", savedOrder.ProductName); } }
Use a Test Database: For integration tests involving databases, it’s recommended to use a test database or an in-memory database to avoid affecting production data.
Best Practices for Integration Testing
- Test in a Controlled Environment: Use test databases and services.
- Keep Tests Maintainable: Avoid overly complex test setups.
- Focus on Key Integrations: Prioritize testing critical integration points.
Scenarios for Integration Testing
- Complex Business Logic: Example: When business processes span multiple modules (e.g., order processing involving inventory, payment, and shipping).
- External System Interactions: Example: When your application interacts with third-party services (e.g., payment gateways, external APIs).
- Database Interactions: Example: When operations involve multiple database tables or transactions (e.g., creating a user profile that affects several related tables).
- Cross-Team Integration: Example: When different teams develop different parts of the application; integration tests ensure the components developed by separate teams work together.
Tools for Integration Testing
Setting Up Integration Testing
To set up integration testing in your project, follow these general steps:
- Install the Hubtel template for integration testing
bash
dotnet new --install Hubtel.Templates.V8.IntegrationTestBasic.Tmpl --nuget-source https://pkgs.dev.azure.com/hubtel/_packaging/hubtel/nuget/v3/index.json --interactive
- Create a new integration test project following the Hubtel standard, using the integration Test Template
- Add the necessary dependencies or packages for the chosen unit testing framework to your project.
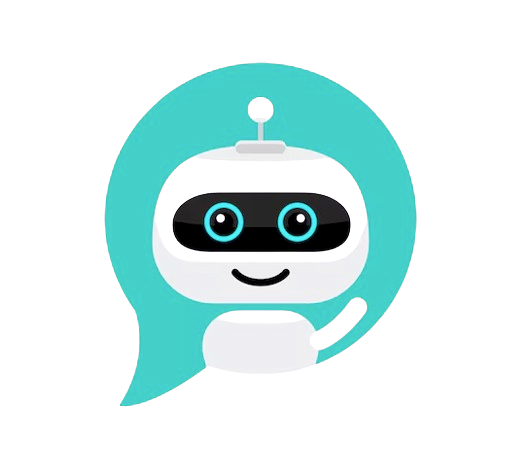
CHAT SAMMIAT