Core Realtime Library Documentation
Overview
The Core Realtime library is designed to facilitate real-time communication between client applications and backend services. It provides an abstraction layer over socket-based communication, making it easier to implement features such as live location tracking, instant messaging, and other real-time updates.
Key Components
1. BaseSocketProvider
This is an abstract class that defines the core functionality for socket communication. It includes methods for:
- Connecting to the socket server
- Emitting events
- Listening to events
- Joining and leaving rooms
2. SocketIOProvider
This class implements the BaseSocketProvider
and uses the Socket.IO client to handle real-time communication. It manages the socket connection and provides methods to interact with the server.
3. SocketData
A generic class that represents the structure of data sent and received through the socket. It includes:
- The actual data payload
- Timestamp
- Source information
- Client ID
4. DataSource
This class contains metadata about the source of the socket data, including:
- Channel
- Client ID
- Platform information
- App details
- OS information
Usage Guide
Initialization
To use the Core Realtime library, start by initializing the SocketIOProvider
:
final socketProvider = SocketIOProvider();
Connecting to the Server
The connection is automatically initiated when the SocketIOProvider
is created. You can check the connection status using:
bool isConnected = socketProvider.isConnected;
Emitting Events
To send data to the server:
final eventId = "update_location";
final data = SocketData<LocationUpdate>(
data: LocationUpdate(latitude: 0.0, longitude: 0.0),
time: DateTime.now().toIso8601String(),
source: DataSource.getDefault(eventId: eventId, clientId: socketProvider.clientId),
);
await socketProvider.emitEvent(eventId, data);
Listening to Events
To receive data from the server:
socketProvider.listenEvent("rider_location", (data) {
// Handle the received data
print("Received location update: $data");
});
Joining and Leaving Rooms
To join a specific room:
socketProvider.joinRoom("delivery_123", null);
To leave a room:
socketProvider.leaveRoom("delivery_123");
Best Practices
- Always check the connection status before performing operations.
- Handle connection errors and disconnects gracefully.
- Use appropriate error handling when emitting events or listening for responses.
- Dispose of the
SocketIOProvider
when it's no longer needed to prevent memory leaks.
Error Handling
The SocketIOProvider
includes error handling for connection issues. You can listen for changes in the connection status by adding a listener to the provider:
socketProvider.addListener(() {
if (!socketProvider.isConnected) {
// Handle disconnection
}
});
Example Use Case: Live Delivery Tracking
Here's how you might use the Core Realtime library for live delivery tracking:
The delivery app joins a room specific to the delivery:
dartsocketProvider.joinRoom("delivery_${orderId}", null);
The app emits location updates:
dartsocketProvider.emitEvent("update_location", SocketData( data: LocationUpdate(latitude: currentLat, longitude: currentLong), time: DateTime.now().toIso8601String(), source: DataSource.getDefault(eventId: "update_location", clientId: socketProvider.clientId), ));
The customer app listens for these updates:
dartsocketProvider.listenEvent("rider_location", (data) { // Update the UI with the new location });
This setup allows for real-time tracking of the delivery rider's location.
Conclusion
The Core Realtime library provides a robust and flexible solution for implementing real-time features in your Flutter applications. By abstracting the complexities of socket communication, it allows developers to focus on building features rather than managing low-level networking details.
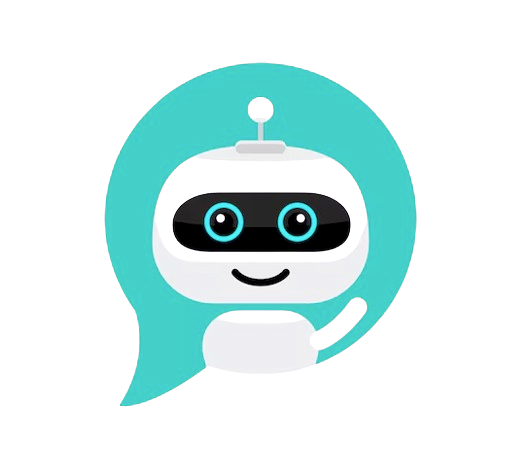
CHAT SAMMIAT