Events Publisher
The Event Publisher is a specialized utility package crafted specifically for frontend applications to capture exceptions and errors. Serving as a conduit, it seamlessly transmits these critical events to a centralized logging subsystem adept at capturing and storing these events as logs.
The Events Portal offers a user-friendly interface within the logging subsystem, empowering real-time search functionalities and comprehensive log analysis. Developers gain access to the Events Portal to actively monitor the performance and health status of their frontend applications, facilitating informed decision-making and proactive issue resolution.
Installation
Copy the snippet below and paste into the
.npmrc
file in your project's root folder. If your project doesn't have a.npmrc
file, create one.txt@hubtel:registry=https://pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/ always-auth=true ; begin auth token //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:username=hubtel //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:_password=YmFibzJ5dHZ4bGRvbWZ0ZHZ5NzZ3czczdG1ueTVqanJnYW0ycTdkeWhpbXRoajNobGxhcQ== //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/registry/:email=npm requires email to be set but doesn't use the value //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:username=hubtel //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:_password=YmFibzJ5dHZ4bGRvbWZ0ZHZ5NzZ3czczdG1ueTVqanJnYW0ycTdkeWhpbXRoajNobGxhcQ== //pkgs.dev.azure.com/hubtel/_packaging/hubtel/npm/:email=npm requires email to be set but doesn't use the value ; end auth token
Run installation command:
bashnpm install
Install Event Publisher:
bashnpm i @hubtel/event-publisher # npm might miss some of the peer dependencies. # You would have to install them independently. npm i ohash
Setting up Event Publisher
Nuxt
Create a composable
useEventPublisher.ts
:tsconst eventPub = new HubtelEventPub({ appBuildNumber: "0.0.1", appId: "partners.hubtel.com", appName: "Partners Portal", appVersion: "0.0.1", eventAnalyticsApiToken: ANALYTICS_EVENTS_TOKEN, // Required for logging analytics events via logAnalyticsEvent }); export default () => { return eventPub; };
Then use it in your components:
useHubtelEvent().logRegularEvent({
//payload
})
Next
- In your
services
folder, create a fileeventPublisher.ts
// eventPublisher.ts
import { HubtelEventPub } from "@hubtel/event-publisher";
const eventPublisher = new HubtelEventPub({
appBuildNumber: "0.0.1",
appId: "admin-payroll.hubtel.com",
appName: "SMB Payroll",
appVersion: "1.0.0",
sectionName: "admin-payroll.hubtel.com",
eventAnalyticsApiToken: ANALYTICS_EVENTS_TOKEN, // Required for logging analytics events via logAnalyticsEvent
isServerApp: false,
});
export default eventPublisher;
- Proceed to use it inside your components:
import eventPublisher from "@/services/eventPublisher";
eventPublisher.logRegularEvent({
// payload
})
Note:
In a Next
app that depends completely or almost completely on React Server Componenets(RSC), add these options to the defaults passed to the constructor
const eventPub = new HubtelEventPub({
appBuildNumber: "0.0.1",
appId: "partners.hubtel.com",
appName: "Partners Portal",
appVersion: "0.0.1",
isServerApp: true // When your app depends almost completely on server components,
userAgent: // provide a userAgent
});
Methods
Event publisher has various methods for logging different kinds of events:
logRegularEvent: Used for logging standard, one-time events that don't require additional tracking beyond the event itself. Typically for simple user actions or system occurrences.
logDurationEvent: Tracks events over a period of time, logging the start and end to calculate duration. Useful for monitoring how long a user spends on an activity or how long a process takes.
logRequestErrorEvent: Captures and log errors that occur during HTTP requests. It helps with tracking and debugging failed network interactions.
logExceptionEvent: Captures exception events, logging details about the failure or unexpected behavior. Useful for monitoring or handling errors and exceptions.
logAnalyticsEvent: Logs specific analytical to gather insights into app usage or performance. This method can be used to log both analytics events and other types of events simultaneously. It serves as a comprehensive option and can ideally replace the use of other specific event logging methods, offering a unified way to track all events and associated analytics.
TIP
The logAnalyticsEvent
method is recommended for logging all event types. It streamlines the process by posting events to both the Events Portal and the analytics endpoint simultaneously, making it more efficient than using individual methods which only post to Events Portal.
Working in Development
When working in a dev environment, events are not logged to the portal but instead in your console. This is to minimize unnecessary logs and activity during development. To test if your setup is working as expected, set developerMode
to false
in the defaults provided to the HubtelEventPub
class. Please note that this is supposed to be for testing your setup and should be cleared immediately after tests.
Was this page helpful?
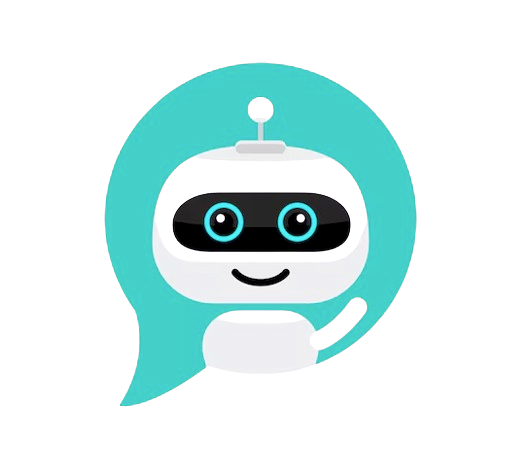
CHAT SAMMIAT