JTACT Device Processor
The JTactProcessor
class is a concrete implementation of the POSDeviceProcessor
interface, specifically designed for processing payments with the JTact POS device. It manages the interaction between the application and the JTact device, handling card reading, EMV transactions, and charging processes.
Properties
- context: The Android
Context
object, used for accessing resources and services. - viewModel: An instance of
ChargeProcessor
, which is responsible for initiating the charging process. - cardProcessResult: A
MutableSharedFlow
that emits results of card processing events. - cardProcessListener: A read-only
SharedFlow
that exposes thecardProcessResult
to listeners. - chargeInfo: An optional
ChargeInfo
object containing details of the charge to be processed. - serialNumber: The serial number of the JTact device.
- cardEntryMode: An enum representing the mode of card entry (swipe, insert, tap).
- transData: A data structure holding transaction data for the current operation.
Methods
- start(chargeInfo: ChargeInfo): Initiates the card processing flow, starting with searching for a card.
- cancel(): Cancels the current card search and emits a cancellation event.
- startCardSearch(readerType: EReaderType): Starts the card search process with the specified reader type.
- onSearchCardResult(searchCardRet: SearchCardRet): Processes the result of the card search.
- processMagSwipe(searchResult: SearchCardRet): Processes a magnetic stripe card transaction.
- processICCRead(searchResult: SearchCardRet): Processes a contactless card transaction.
- processPICCRead(searchResult: SearchCardRet): Processes an inserted card transaction.
The start
Method
The start
method is a suspending function that initiates the card processing workflow for the JTact POS device. It sets up the initial state, retrieves the device serial number, and begins the search for a card.
Flow of Execution
- The method assigns the provided
chargeInfo
to the class propertythis.chargeInfo
. - It retrieves the device serial number and logs it.
- It emits a
CardProcessResult.Message
with thechargeInfo.message
to inform the user of the current status. - It starts the card search process with the specified reader types.
- Depending on the result of the card search, it either processes the card information or emits an error.
Callback Implementations
- onSearchCardResult(searchCardRet: SearchCardRet): This callback is triggered when card information is successfully retrieved. It determines the card entry mode and updates the
transData
accordingly. Then, it processes the card based on the entry mode.
Companion Object Constants
- TAG: Logging tag for the class.
Usage
To use the JTactProcessor
class, create an instance of the class and call the start
method with a ChargeInfo
object that contains the details of the charge to be processed. The class will manage the card reading process, EMV transactions, and emit results through the cardProcessListener
shared flow.
val jTactProcessor = JTactProcessor(context)
val chargeInfo = // ... obtain or create a ChargeInfo instance
launch {
jTactProcessor.start(chargeInfo)
}
This documentation provides an overview of the JTactProcessor
class, its properties, methods, and usage for processing payments with the JTact POS device.
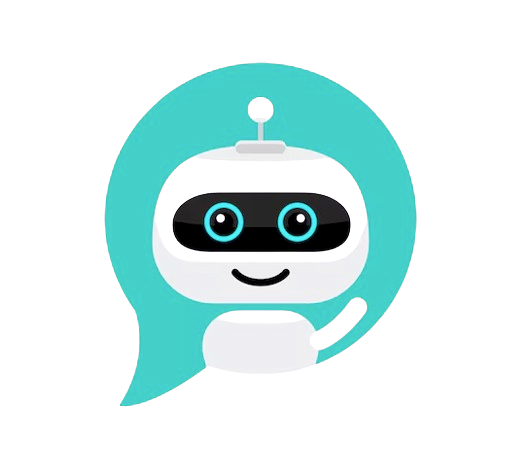
CHAT SAMMIAT