Test Parallelization
Vitest supports parallel test execution, a feature that allows multiple tests to run simultaneously, reducing the total time it takes to complete all tests. This is particularly beneficial in large projects with many tests or tests that have long wait times due to network requests, timeouts, or heavy computations.
Using describe.concurrent
In Vitest, the describe.concurrent
method is used to mark a block of tests that can be run in parallel. This is an alternative to the standard describe
block, which runs its tests sequentially. Here’s how it works:
Syntax:
describe.concurrent
is used just like the regular describe function, but it tells Vitest that the tests inside this block should be executed in parallel.Execution : Tests within a
describe.concurrent
block are started at the same time. Each test runs independently, and the completion of one test does not affect the others.
Here's a sample code breakdown:
//parallel.spec.ts
import { describe, it } from "vitest";
const sleep = (time = 1000) => {
return new Promise<void>((resolve) => {
setTimeout(() => {
resolve();
}, time);
});
};
describe.concurrent("sleep", () => {
it("should sleep for 500ms", async ({ expect }) => {
await sleep(500);
expect(true).toBe(true);
});
it("should sleep for 750ms", async ({ expect }) => {
await sleep(750);
expect(true).toBe(true);
});
it("should sleep for 1000ms", async ({ expect }) => {
await sleep(1000);
expect(true).toBe(true);
});
it("should sleep for 1500ms", async ({ expect }) => {
await sleep(1500);
expect(true).toBe(true);
});
});
Functionality: The
sleep
function is an asynchronous function that returns a promise that resolves after a specified timeout period. This function is used to simulate delayed operations.Test Cases: Each test within the
describe.concurrent
block waits for a different duration (500ms, 750ms, 1000ms, and 1500ms). Despite these differing wait times, all tests are initiated simultaneously.Assertions: Each test simply asserts true to be true after the sleep duration. This assertion is trivial and always true; it acts as a placeholder in this example. Typically, you would replace this with meaningful assertions that check the effects or outcomes of what you are actually testing (e.g., changes in state, effects on other components, etc.).
Best Practices for Parallelization
Use Where Appropriate: While parallelization can speed up the execution of tests, it is best used for tests that are self-contained and do not interfere with each other. Avoid using it for tests that share state or resources.
Resource Management: Ensure that your testing environment can handle multiple tests running in parallel without running out of resources or causing bottlenecks.
Debugging: Be aware that debugging parallel tests can be more challenging than sequential tests, as concurrent operations can lead to non-deterministic behaviors.
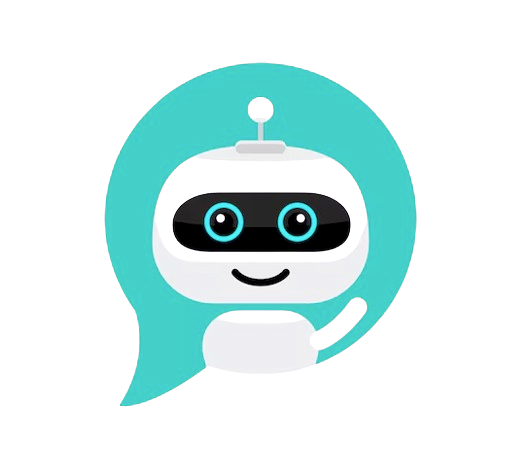
CHAT SAMMIAT