Z90 Device Processor
The Z90Processor
class is a concrete implementation of the POSDeviceProcessor
interface, tailored for processing payments with the Z90 POS device. It manages the interaction between the application and the Z90 device, handling card reading, EMV transactions, and charging processes.
Properties
- context: The Android
Context
object, used for accessing resources and services. - chargeProcessor: An instance of
ChargeProcessor
, which is responsible for initiating the charging process. - cardProcessResult: A
MutableSharedFlow
that emits results of card processing events. - track2Str: A string representing the Track 2 data from the card.
- cardReaderManager: Lazily initialized instance of the card reader manager from the
DriverManager
. - pinPadManager: Lazily initialized instance of the pin pad manager from the
DriverManager
. - beeper: Lazily initialized instance of the beeper from the
DriverManager
. - emvHandler: Singleton instance of
EmvHandler
for handling EMV transactions. - icInsertCard: Instance of
ICCard
for handling IC card transactions. - magSwipeCard: Instance of
MagCard
for handling magnetic stripe card transactions. - rfTapCard: Instance of
RfCard
for handling contactless card transactions. - cardEntryMode: Enum representing the mode of card entry (insert, swipe, tap).
- cardInfoEntity: Entity holding information about the processed card.
- cardData: Data structure holding detailed card information.
- realCardType: Enum representing the actual type of the card being processed.
- mLatch: A
CountDownLatch
used for synchronizing the input PIN process. - inputPINResult: Result code from the input PIN process.
- pinBlock: Byte array for storing the PIN block.
- expiryDate: Expiration date of the card.
- chargeInfo: Information about the charge to be processed.
- serialNumber: Serial number of the Z90 device.
Methods
- start(chargeInfo: ChargeInfo): Initiates the card processing flow, starting with searching for a card.
- cancel(): Cancels the current card search and emits a cancellation event.
- cancelCardSearch(): Cancels the card search process.
- onCardInfoInternal(cardInfoEntity: CardInfoEntity?): Processes the card information internally based on the card type.
- processRFCard(rfCardType: Byte): Processes a contactless card transaction.
- processMagCard(): Processes a magnetic stripe card transaction.
- processICCard(): Processes an inserted card transaction.
- initEmv(cardType: CardReaderTypeEnum?): Initializes the EMV transaction parameters and processes the EMV transaction.
- inputPIN(pinType: Byte): Handles the input of the PIN for EMV transactions.
The start
Method
The start
method is a suspending function that initiates the card processing workflow for the Z90 POS device. It is designed to be called from a coroutine context and is responsible for setting up the initial state, canceling any ongoing card search, and beginning the search for a card.
Parameters
- chargeInfo: An instance of
ChargeInfo
that contains the details of the charge to be processed, including the message to be displayed to the user.
Flow of Execution
- The method begins by assigning the provided
chargeInfo
to the class propertythis.chargeInfo
. - It calls
cancelCardSearch()
to halt any ongoing card search process. - It launches a coroutine to emit a
CardProcessResult.Message
with thechargeInfo.message
to inform the user of the current status. - It invokes
cardReaderManager.searchCard
to begin searching for a card. The search is configured to look for magnetic, inserted, or contactless cards (CardReaderTypeEnum.MAG_IC_RF_CARD
) and has a timeout period defined byREAD_TIME_OUT
. - The
searchCard
method accepts anOnSearchCardListener
object, which defines callbacks for when card information is available, an error occurs, or no card is detected.
Callback Implementations
- onCardInfo(cardInfoEntity: CardInfoEntity?): This callback is triggered when card information is successfully retrieved. It emits a
CardProcessResult.Message
indicating that the card is being searched and then callsonCardInfoInternal(cardInfoEntity)
to process the card information internally. - onError(err: Int): This callback is invoked when an error occurs during the card search. It emits a
CardProcessResult.Error
with a generic reading error message. - onNoCard(p0: CardReaderTypeEnum?, p1: Boolean): This callback is called when no card is detected. The current implementation is empty, indicating that no action is taken when no card is present.
Companion Object Constants
- ASSETS_PATH: Path to the assets directory for EMV parameter files.
- READ_TIME_OUT: Timeout value for reading card information.
- TAG: Logging tag for the class.
- Error_FREQ: Frequency for the beeper when an error occurs.
- ERROR_TIME: Duration for the beeper when an error occurs.
- FOUND_FREQ: Frequency for the beeper when a card is found.
- FOUND_TIME: Duration for the beeper when a card is found.
Usage
To use the Z90Processor
class, create an instance of the class and call the start
method with a ChargeInfo
object that contains the details of the charge to be processed. The class will manage the card reading process, EMV transactions, and emit results through the cardProcessListener
shared flow.
val z90Processor = Z90Processor(context)
val chargeInfo = // ... obtain or create a ChargeInfo instance
launch {
z90Processor.start(chargeInfo)
}
This documentation provides an overview of the Z90Processor
class, its properties, methods, and usage for processing payments with the Z90 POS device.
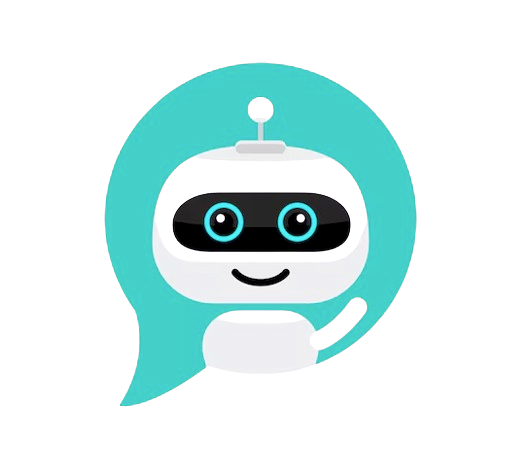
CHAT SAMMIAT