Getting Started with Hubtel Obsidian SDK
This SDK helps you to create , update and delete job schedules in obsidian.
In order to get started with Hubtel.Obsidian.Sdk, do the following activities:
Install-Package Hubtel.Obsidian.Sdk
The provided code snippet is a command that is used to install Hubtel.Obsidian.Sdk
package in a .NET project.
Install-Package Hubtel.Obsidian.Sdk
Add to appsettings.json
The "HubtelObsidianConfig"
block contains several properties:
"Url"
: This property specifies the URL of the Obsidian service. In this case, it's set to a local development server running on port 8080."Username"
: This property specifies the username used for authentication with the Obsidian service. Here, it's set to "admin"."Password"
: This property specifies the password used for authentication with the Obsidian service. Here, it's set to "changeme"."Timeout"
: This property specifies the timeout duration in seconds for requests to the Obsidian service. Here, it's set to 45 seconds.
"HubtelObsidianConfig": {
"Url" : "http://localhost:8080/rest/jobs/",
"Username" : "admin",
"Password" : "changeme",
"TImeout" : 45
}
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelObsidianSdk(c => {...});
This line is adding the Hubtel Obsidian SDK to the application's services. This is presumably a method provided by the
Hubtel.Obsidian.Sdk
package that sets up any necessary services for the SDK to function, such as HTTP clients or other dependencies.The
AddHubtelObsidianSdk
method takes a lambda expression as an argument, which configures the SDK settings:c.Url = Configuration["HubtelObsidianConfig:Url"]
: Sets the URL of the Obsidian service.c.Username = Configuration["HubtelObsidianConfig:Username"]
: Sets the username used for authentication with the Obsidian service.c.Password = Configuration["HubtelObsidianConfig:Password"]
: Sets the password used for authentication with the Obsidian service.c.Timeout = int.Parse(Configuration["HubtelObsidianConfig:Timeout"])
: Sets the timeout duration in seconds for requests to the Obsidian service.The Configuration
object is used to retrieve these settings from the application's configuration, which is typically stored in a file likeappsettings.json
.
services.AddHubtelObsidianSdk(c =>
{
c.Url = Configuration["HubtelObsidianConfig:Url"];
c.Username = Configuration["HubtelObsidianConfig:Username"];
c.Password = Configuration["HubtelObsidianConfig:Password"];
c.Timeout = int.Parse(Configuration["HubtelObsidianConfig:Timeout"]);
});
Inject and consume service methods
private readonly IObsidianClient _obsidianClient;
- This line declares a private, read-only field named
_obsidianClient
of typeIObsidianClient
. This is presumably an interface provided by the Hubtel Obsidian SDK for interacting with the Obsidian service.
The following lines of code demonstrate how to create, update, and delete job schedules using the _obsidianClient
:
var request = ObsidianRequest.CreateRequest("test3444", "http://localhost:7989", DateTime.Now.AddDays(7))
- This line creates a new
ObsidianRequest
object with a job name, a callback URL, and a date when the job should be executed.
var response = await _obsidianClient.CreateJob(request);
- This line sends the ObsidianRequest to the Obsidian service to create a new job schedule. The response from the service is stored in the
response
variable.
var response = await _obsidianClient.UpdateJob(new ObsidianUpdateRequest {...}, "205");
- This line sends an
ObsidianUpdateRequest
to the Obsidian service to update an existing job schedule. The job schedule to update is identified by the string "205". TheObsidianUpdateRequest
object specifies the new schedule, state, effective date, and end date for the job.
var response = await _obsidianClient.DeleteJob("203");
- This line sends a request to the Obsidian service to delete an existing job schedule. The job schedule to delete is identified by the string "203".
private readonly IObsidianClient _obsidianClient;
//To Create a Job Schedule
var request = ObsidianRequest.CreateRequest("test3444", "http://localhost:7989", DateTime.Now.AddDays(7));
var response = await _obsidianClient.CreateJob(request);
//To Update a Job Schedule
var response = await _obsidianClient.UpdateJob(new ObsidianUpdateRequest
{
Schedule = "19 * * 9 *",
State = "ENABLED",
EffectiveDate = "2021-01-15T16:19:00+0000",
EndDate = "2021-01-30T20:17:00+0000"
}, "205");
//To Delete a Job Schedule
var response = await _obsidianClient.DeleteJob("203");
Was this page helpful?
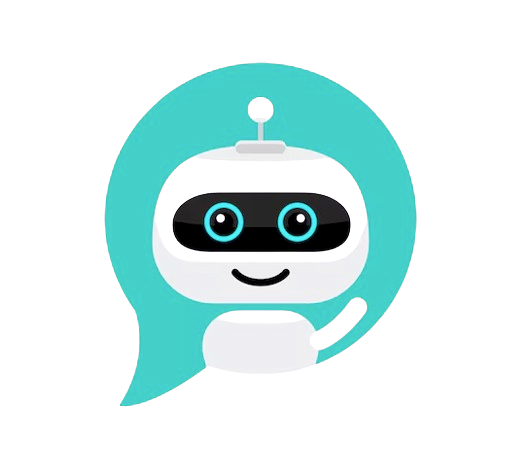
CHAT SAMMIAT