Skip to content
Getting Started with Bogus
Bogus is a useful .NET library used for generating fake/mock data for testing, prototyping, or populating databases.
In order to get started with Bogus, do the following activities
Installing Bogus Package
Install-Package Bogus
Configuration
Create Faker instance and Generate "fake" data
After installing the Bogus package, create a Faker instance and configure it to generate data for your desired class parameters.
Sample Usage
This is a sample use case to see how it is works:
Class to generate data for
c#
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
Create and configure Faker instance
c#
var faker = new Faker<Person>()
.RuleFor(p => p.Name, f => f.Person.FullName)
.RuleFor(p => p.Age, f => f.Person.Age);
Generate fake data
c#
var fakePerson = faker.Generate();
string Name = fakePerson.Name;
int Age = fakePerson.Age;
You can also generate multiple fake instances of a class:
c#
var fakePeople = faker.Generate(5);//generates five instances with fake data
foreach (var person in fakePeople) //Iterating through generated data and displaying on console
{
Console.WriteLine($"Name: {person.Name}");
Console.WriteLine($"Age: {person.Age}");
}
Further Readings
Follow this link to learn more.
Was this helpful? 📚
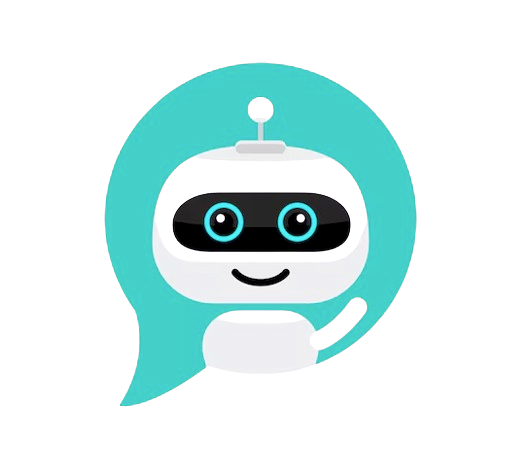
CHAT SAMMIAT