Skip to content
Getting Started with Refit
Refit is a library for generating type-safe HTTP clients in .NET. It allows you to turn your API into a live interface with annotated methods that represent your API endpoints! Refit then generates an implementation of that interface at runtime using your HTTPClient, providing a simple and type-safe way to make HTTP requests and handle responses.
In order to get started with Refit, do the following activities
Installing Refit Package
Install-Package Refit
Configuration
Add HttpClient with base URL in Startup or Configuration class
After installing the Refit package, it can be configured to use a specific HTTP client implementation in your Startup or Configuration class as follows:
c#
services.AddHttpClient("MyApiClient", client =>
{
client.BaseAddress = new Uri("https://api.example.com/");//Replace with your base URL
});
Add Refit API interface with HttpClient in Startup or Configuration class
c#
services.AddRefitClient<IMyApi>() //Replace with the name of your API interface
.ConfigureHttpClient(c => c.DefaultRequestHeaders.Add("ApiKey", "YOUR_API_KEY"))//Replace with API header name and API key value
.SetHandlerLifetime(TimeSpan.FromMinutes(10)) // Adjust the lifetime as needed
.AddHttpMessageHandler<YourCustomHandler>(); // Add any custom handlers if required
Sample Usage
After installing Refit and configuring with your HTTP client, this is a sample use case to see how it works.
Define API Interface representing API endpoints
c#
public interface IMyApi
{
[Get("/users/{id}")]
Task<User> GetUser(string id);
[Post("/users")]
Task<User> CreateUser([Body] User user);
[Put("/users/{id}")]
Task<User> UpdateUser(string id, [Body] User user);
[Delete("/users/{id}")]
Task DeleteUser(string id);
}
Inject API interface and consume API enpoint methods in Services or anywhere in your code!
c#
public class UserService
{
private readonly IMyApi _myApi;
public UserService(IMyApi myApi)
{
_myApi = myApi;
}
public async Task<User> GetUser(string id)
{
return await _myApi.GetUser(id);
}
public async Task<User> CreateUser(User user)
{
return await _myApi.CreateUser(user);
}
public async Task<User> UpdateUser(string id, User user)
{
return await _myApi.UpdateUser(id, user);
}
public async Task DeleteUser(string id)
{
await _myApi.DeleteUser(id);
}
}
Further Readings
Follow this link to learn more.
Was this helpful? 📚
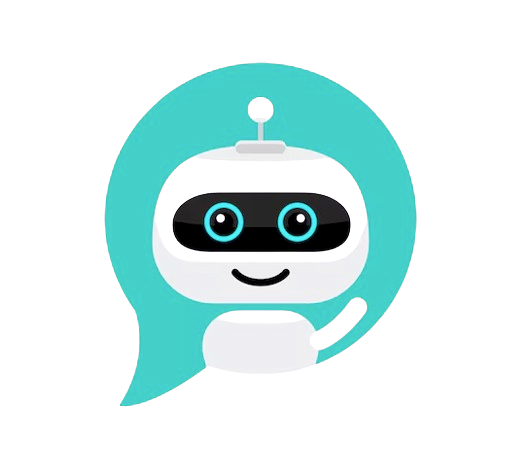
CHAT SAMMIAT