Core Utils Library Documentation
Overview
The Core Utils Library is a comprehensive collection of utility functions, extensions, and helper classes designed to streamline development in Flutter applications. It provides a wide range of tools for common tasks such as string manipulation, date formatting, device information retrieval, and more.
Key Components
1. Extensions
The library offers various extension methods to enhance the functionality of built-in Dart types:
- String Extensions: Additional methods for string manipulation and validation.
- Currency Extensions: Formatting and handling of currency-related operations.
- Date Extensions: Parsing, formatting, and manipulating dates.
- Event Metadata Extensions: Handling of analytics event metadata.
2. Helpers
A collection of helper classes to simplify common tasks:
- DeviceHelper: Retrieve device-specific information.
- HapticFeedbackHelper: Easily trigger haptic feedback.
- IdGeneratorHelper: Generate unique identifiers.
- MoneyTextInputFormatter: Format currency input in text fields.
- PermissionHandler: Manage and request app permissions.
3. Models
Utility models and interfaces for structuring data:
- Serializable: Interface for objects that can be serialized to JSON.
- Partitionable: Interface and extensions for grouping data.
- Searchable: Interface and extensions for implementing search functionality.
4. Analytics
Utilities for handling analytics events:
- AnalyticsEvent: Base class for defining analytics events.
- EventMetadata: Structure for additional event metadata.
Usage
String Extensions
String? nullableString = null;
bool isBlank = nullableString.isNullOrBlank(); // true
String url = "example.com/api".appendToUrl("users", "profile");
// Result: "example.com/api/users/profile"
Device Information
DeviceInfo deviceInfo = await DeviceHelper.getDeviceInfo();
print(deviceInfo.osVersion);
print(deviceInfo.manufacturer);
Money Formatting
final moneyFormatter = MoneyTextInputFormatter(
allowFraction: true,
decimalPlaces: 2,
);
TextField(
inputFormatters: [moneyFormatter],
keyboardType: TextInputType.number,
);
Searchable Interface
class MySearchableItem implements Searchable {
@override
List<String> get searchableTerms => ['term1', 'term2'];
}
List<MySearchableItem> items = [/* ... */];
var filteredItems = items.filterWithSearchableTerms(searchTerm: 'term');
Permissions
class MyClass with PermissionHandler {
Future<void> requestCameraPermission() async {
bool granted = await requestPermission(Permission.camera);
if (granted) {
// Camera permission granted
}
}
}
Best Practices
- Utilize the provided extensions and helpers to maintain consistency across your application.
- Implement the Serializable interface for objects that need to be converted to JSON.
- Use the Searchable interface and extensions for implementing efficient search functionality.
- Leverage the DeviceHelper for retrieving device-specific information when needed.
- Implement the Partitionable interface for easy grouping and sectioning of data.
Conclusion
The Core Utils Library provides a robust set of utilities and helpers that can significantly streamline Flutter development. By leveraging these tools, developers can write more efficient, readable, and maintainable code while reducing boilerplate and repetitive tasks.
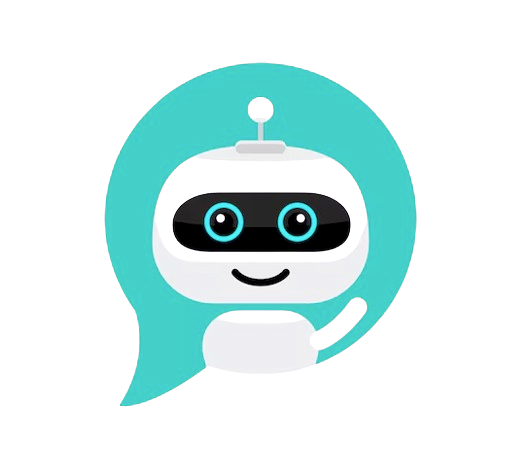
CHAT SAMMIAT