Integrating Hubtel Internal SDK's in Flutter
This guide explores the process of integrating internal SDKs (Software Development Kits) into Flutter applications. Internal SDKs often reside in private repository projects, and their inclusion involves setting up secure connections, leveraging SSH authentication, and ensuring seamless integration within your Flutter codebase. By following this guide, developers can efficiently incorporate proprietary functionalities, streamline collaboration on closed-source projects, and maintain a robust and secure development workflow within their organization.
To integrate an internal package in your Flutter project, you'll need to do the following steps:
Generate an SSH Key Pair
- In your project run the shell command
./get_ssh_key.sh
, this file should be located in the root of your project. However if it isn't there you can copy the code below and save it in a file named get_ssh_key.sh at the root of your project.
#!/bin/bash
echo "Running SSH key generation..."
# Define the path to the SSH key
SSH_KEY_PATH="$HOME/.ssh/id_rsa"
# Check if the SSH key already exists
if [ ! -f "$SSH_KEY_PATH" ]; then
echo "SSH key not found, generating one..."
# Generate the SSH key
ssh-keygen -t rsa -b 2048 -f "$SSH_KEY_PATH" -N ''
else
echo "SSH key already exists."
fi
echo ""
# Read and print the public key
echo "Your public SSH key is:"
cat "${SSH_KEY_PATH}.pub"
# Print instructions for the user
echo ""
echo "Copy the public key above to your clipboard."
echo "Follow the instructions at http://bit.ly/3SotbOH to add this key to your server."
Copy the public key provided in your terminal and add it to your Azure DevOps account's SSH keys.
Open your security settings by browsing to the web portal and selecting the icon next to the avatar in the upper right of the user interface. Select
SSH public keys
in the menu that appears.Select
+ New Key
Paste the copied public key into the
Public Key Data
field and set the Name asHubtel-Internal-SDK-Token
. Avoid adding whitespace or new lines into theKey Data
field, as they can cause Azure DevOps to use an invalid public key. When pasting in the key, a newline often is added at the end. Be sure to remove this newline if it occurs.Once added, you can't change the key. You can delete the key or create a new entry for another key. There are no restrictions on how many keys you can add to your user profile. Also note that SSH keys stored in Azure DevOps expire after one year. If your key expires, you may upload a new key or the same one to continue accessing Azure DevOps via SSH.
On the overview page a note is displayed at the top containing the server fingerprints. Make note of them because they will be required when you first connect to Azure DevOps via SSH.
Finally test the connection running the following command:
ssh -T [email protected]
If this was the first time connecting you should receive the following output:
The authenticity of host 'ssh.dev.azure.com (<IP>)' can't be established.
RSA key fingerprint is SHA256:ohD8VZEXGWo6Ez8GSEJQ9WpafgLFsOfLOtGGQCQo6Og.
This key is not known by any other names
Are you sure you want to continue connecting (yes/no/[fingerprint])?
Compare the given fingerprint with the fingerprints offered on the settings page. Proceed only if they match!
If everything is configured correctly the output should look like this:
remote: Shell access is not supported.
This means that the connection was successful.
Add the Internal SDK as a Dependency in Flutter
In your Flutter application, add the Azure Repo as a dependency in your pubspec.yaml
by specifying the SSH URL. To get the SSH URL, navigate to the Azure DevOps repository and select the Clone
button. Choose the SSH option and copy the URL.
In your flutter project and the line below to your pubspec.yaml
file and replace the url with the one you copied from azure and provide how you want to reference the package, using a tag or a branch name. Also replace the package_name
dependencies:
<package_name>:
git:
url: [email protected]:v3/hubtel/Mobile-Apps/Platform-Library-Flutter-Hubtel-Auth
ref: <tag version> or <branch>
Example of a pubspec.yaml
file including the core platform package:
dependencies:
core_platform:
git:
url: [email protected]:v3/hubtel/Mobile-Apps/Platform-Library-Flutter-Core-Platform
ref: 0.0.11
Run flutter pub get
Execute flutter pub get in your application directory to fetch the package from the Azure Repo.
flutter pub get
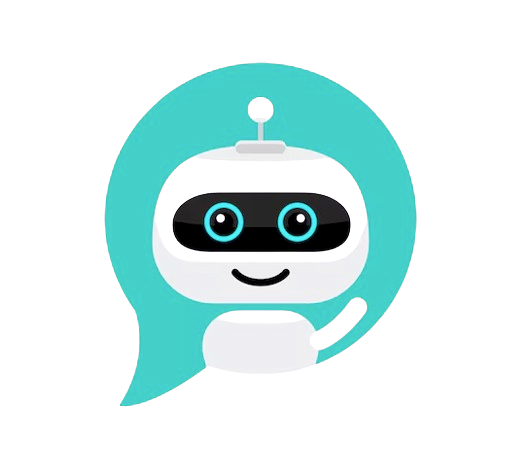
CHAT SAMMIAT