Getting Started with Fineract API SDK
This SDK helps you to interact with an instance of the API. Fineract is a platform for digital financial services, particularly microfinance and digital banking. It's an open-source system provided by the Apache Software Foundation. Fineract provides a reliable, robust, and affordable solution for entrepreneurs, financial institutions, and service providers to offer financial services to the world’s 2 billion underbanked and unbanked.
The Fineract API SDK would provide developers with a set of tools and libraries that simplify the process of interacting with the Fineract API, making it easier to build applications that leverage Fineract's capabilities.
In order to get started with Hubtel.Fineract.Sdk, do the following activities
Install-Package Hubtel.Fineract.Sdk
The command below is used in the NuGet Package Manager Console in Visual Studio. This command is written in PowerShell and it's used to install Hubtel.Fineract.Sdk
into the current .NET project.
Install-Package Hubtel.Fineract.Sdk
The FineractConfiguration
object contains several properties:
BaseUrl
: The base URL of the Fineract API. All API endpoints will be relative to this URL.Username
: The username used to authenticate with the Fineract API.Password
: The password used to authenticate with the Fineract API.ClientRoute
: The API route for interacting with clients. This is appended to the BaseUrl to form the full URL for client-related requests.SavingsAccountRoute
: The API route for interacting with savings accounts.SavingsProductRoute
: The API route for interacting with savings products.ApproveSavingsAccountRoute
: The API route for approving savings accounts.CheckAccountBalanceRoute
: The API route for checking account balances.TransferFundsRoute
: The API route for transferring funds between accounts.TenantIdentifier
: The identifier for the tenant. In a multi-tenant system like Fineract, this is used to separate data between different tenants.This configuration allows the application to connect to the Fineract API, authenticate using the provided username and password, and interact with various parts of the system by making requests to the specified routes.You will need to provide the following configuration values in the
appsettings.json
file:
"FineractConfiguration": {
"BaseUrl": "<base_url>",
"Username": "<username>",
"Password": "<password>",
"ClientRoute": "clients",
"SavingsAccountRoute": "savingsaccounts",
"SavingsProductRoute": "savingsproducts",
"ApproveSavingsAccountRoute": "savingsaccounts",
"CheckAccountBalanceRoute": "accounts/check-balance",
"TransferFundsRoute": "accounttransfers",
"TenantIdentifier": "default"
}
Add to IServiceCollection in Program.cs or Startup.cs
services.AddHubtelFineractSdk
is a method call that adds the Hubtel Fineract SDK to the application's services. This makes the SDK available for dependency injection throughout the application.The argument to
AddHubtelFineractSdk
is a lambda expression that configures the SDK. This lambda expression uses theConfiguration
object to get theFineractConfiguration
section from the application's configuration (usually defined inappsettings.json
).Configuration.GetSection(nameof(FineractConfiguration))
retrieves theFineractConfiguration
section from the application's configuration. Thenameof
keyword is used to avoid hard-coding the string "FineractConfiguration"..Bind(o)
binds the configuration values to the FineractConfiguration object. This means that the properties of the FineractConfiguration object will be set to the corresponding values from the configuration.
services.AddHubtelFineractSdk(o => Configuration.GetSection(nameof(FineractConfiguration)).Bind(o));
Inject and consume service methods
readonly
means that the value of this field can only be set at the time of declaration or within the constructor of the same class. It cannot be changed afterwards. This is useful for dependencies that should not change once they are set.IFineractService
is an interface that presumably provides methods for interacting with the Fineract API. The actual implementation of this interface would contain the logic for making API calls to Fineract._fineractService
is the instance of theIFineractService
that will be used within the class. This instance would typically be provided through dependency injection, which is a technique that allows the system to provide dependencies to a class, rather than the class having to construct them itself.This line of code is declaring a dependency on a service that provides functionality related to the Fineract API. This service is provided to the class through dependency injection, and once set, it cannot be changed.
private readonly IFineractService _fineractService;
Create Savings Account
This method creates a savings account for a client in the Fineract API.
A
CreateSavingsAccountRequestDto
object that contains the details of the savings account to be created.
public async Task<ApiResponse<CreateSavingsAccountResponseDto>> CreateSavingsAccount(CreateSavingsAccountRequestDto createSavingsDto)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
CreateSavingsAccountResponseDto
object that contains the details of the savings account that was created, or null if the operation failed.
Create Client
This method creates a client account in the Fineract API.
A
CreateClientRequestDto
object that contains the details of the client account to be created.
public async Task<ApiResponse<CreateClientResponseDto>> CreateClientAccount(CreateClientRequestDto createClientDto)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
CreateSavingsAccountResponseDto
object that contains the details of the savings account that was created, or null if the operation failed.
Create Savings Product
This method creates a savings product in the Fineract API.
A
CreateSavingsProductRequestDto
object that contains the details of the client account to be created.
public async Task<ApiResponse<CreateSavingsProductResponseDto>> CreateSavingsProduct(CreateSavingsProductRequestDto createDto)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
CreateSavingsAccountResponseDto
object that contains the details of the savings account that was created, or null if the operation failed.
Transfer Funds
This method transfer funds from on account to another in the Fineract API.
A
TransferFundsRequestDto
object that contains the details of the client account to be created.
public async Task<ApiResponse<TransferFundsResponseDto>> TransferFunds(TransferFundsRequestDto transferFundsDto)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
TransferFundsResponseDto
object that contains the details of the savings account that was created, or null if the operation failed.
Approve or disapprove savings account
This method either approves or disapprove in the Fineract API.
A
ApproveSavingsAccountRequestDto
object that contains the details of the client account to be created.
public async Task<ApiResponse<ApproveSavingsAccountResponseDto>> ApproveSavingsAccount(ApproveSavingsAccountRequestDto approveAccountDto)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
ApproveSavingsAccountResponseDto
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve client by Id
This method retrieves client by Id in the Fineract API.
An
id
object that contains the details of the client account to be created.
public async Task<ApiResponse<ClientsModel>> GetClients(string id)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
ClientsModel
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve clients
This method retrieves clients in the Fineract API.
A
ClientQueryModel
object that contains the details of the client account to be created.
public async Task<ApiResponse<QueryResponseModel<ClientsModel>>> GetClients(ClientQueryModel queryModel)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
QueryResponseModel<ClientsModel>
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve savings account by Id
This method retrieves savings account by Id in the Fineract API.
An
id
object that contains the details of the client account to be created.
public async Task<ApiResponse<SavingsAccountModel>> GetClients(string id)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
SavingsAccountModel
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve savings accounts
This method retrieves savings accounts in the Fineract API.
A
SavingsAccountQueryModel
object that contains the details of the client account to be created.
public async Task<ApiResponse<QueryResponseModel<SavingsAccountModel>>> GetClients(SavingsAccountQueryModel queryModel)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
QueryResponseModel<SavingsAccountModel>
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve savings product by Id
This method retrieves savings product by Id in the Fineract API.
An
id
object that contains the details of the client account to be created.
public async Task<ApiResponse<SavingsProductModel>> GetClients(string id)
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
SavingsProductModel
object that contains the details of the savings account that was created, or null if the operation failed.
Retrieve savings products
- This method retrieves savings product in the Fineract API.
public async Task<ApiResponse<QueryResponseModel<SavingsAccountModel>>> GetClients()
Returns:
An ApiResponse object that contains the following properties:
Code: An HTTP status code that indicates the result of the operation.
Message: A message that describes the result of the operation.
Data: A
QueryResponseModel<SavingsProductModel>
object that contains the details of the savings account that was created, or null if the operation failed.
Error Handling
In case of an error, the API returns a response with an error message and an HTTP status code. The response body has the following format:
{
"code": <HTTP Status Code>,
"message": "<Error Message>",
"data": null
}
Was this helpful? 📚
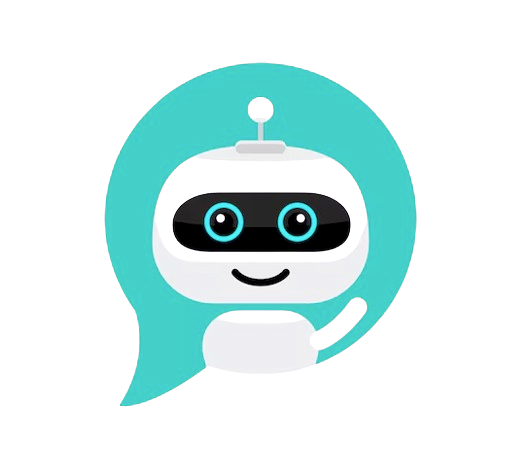
CHAT SAMMIAT