Z90 Printer
The Z90Printer
class is a concrete implementation of the POSPrinter
interface specifically designed for the Z90 POS device. It provides functionality to print receipts or other documents on the Z90 device using the printer driver provided by the DriverManager
.
Properties
printer
: A lazily initialized property that holds the printer instance obtained from theDriverManager
.
Methods
print(creator: ReceiptCreator)
: A suspending function that prints a receipt created by the providedReceiptCreator
. This function is designed to be called from a coroutine context.
print
Method Details
The print
method performs the following steps:
- Builds a bitmap representation of the receipt using the
ReceiptCreator
'sbuild()
method. - Sets the alignment for appending the bitmap to the printer buffer to center.
- Creates a
PrnStrFormat
object with centered alignment and bold style for formatting strings. - Appends a space and a newline character to the printer buffer using the formatted style.
- Starts the print job and checks the result.
- Based on the result of the print job, it either resumes the coroutine with an appropriate exception or proceeds with the next steps.
Exception Handling
The method includes exception handling for common printer issues such as:
- Out of Paper: Throws an exception indicating that the printer is out of paper.
- General Error: Throws an exception indicating a general error during printing.
- Overheating: Throws an exception indicating that the printer is overheating.
- Fault: Throws an exception indicating a fault with the printer.
Usage
To use the Z90Printer
class, create an instance of the class and call the print
method with a ReceiptCreator
object that defines the content to be printed. This method should be called from a coroutine scope to ensure proper asynchronous execution.
val z90Printer = Z90Printer()
val receiptCreator = // ... obtain or create a ReceiptCreator instance
launch {
z90Printer.print(receiptCreator)
}
This documentation provides an overview of the Z90Printer
class, its properties, methods, and exception handling for printing tasks on the Z90 POS device.
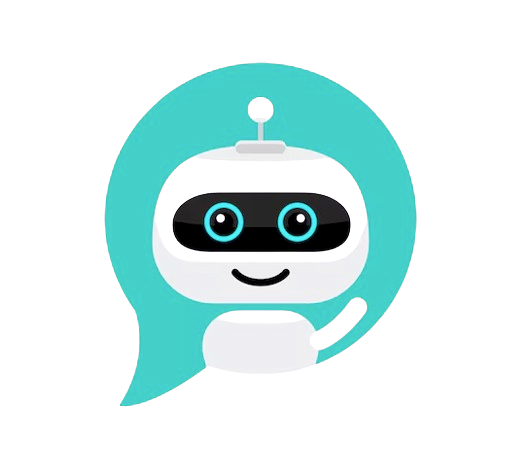
CHAT SAMMIAT