Getting Started with Orders SDK
This SDK helps you to create and order via Order Processor API.
In order to get started with Hubtel.Orders.Sdk, do the following activities:
Install-Package Hubtel.ConsumerData.Sdk
The command below is used in the NuGet Package Manager Console in Visual Studio. This command is written in PowerShell and it's used to install Hubtel.Orders.Sdk
package into the current .NET project.
Install-Package Hubtel.Orders.Sdk
Add to appsettings.json
The OrdersApiSdkConfig
object contains several properties:
CreateOrderUrl
: The URL endpoint for creating new orders. This is where the application will send POST requests to create new orders.AuthKey
: The authentication key used to authenticate with the Orders API. This could be a basic authentication key or another form of API key.OrdersBaseUrl
: The base URL of the Orders API. All API endpoints will be relative to this URL.SavedOrderUrl
: The URL endpoint for interacting with saved orders. This could be used to retrieve, update, or delete saved orders.BearerAuthKey
: The bearer token used for authentication. Bearer tokens are a type of access token that allows the client to access the API on behalf of a user.This configuration allows the application to connect to the Orders API, authenticate using the provided keys, and interact with various parts of the system by making requests to the specified URLs.
"OrdersApiSdkConfig": {
"CreateOrderUrl": "http://order-processor-URL-here/api/v2/orders",
"AuthKey": "auth key here",
"OrdersBaseUrl": "http://order-api-URL-here/api/v1",
"SavedOrderUrl": "http://saved-orders-api-URL-here/api/v1/savedorders",
"BearerAuthKey": "bearer-token-here"
}
Add to IServiceCollection in Program.cs or Startup.cs
This code is configuring the OrdersApiSdkConfig
object with the values from the application's configuration (usually defined in appsettings.json
). Configuration.GetSection(nameof(OrdersApiSdkConfig))
retrieves the OrdersApiSdkConfig
section from the application's configuration. The nameof
keyword is used to avoid hard-coding the string "OrdersApiSdkConfig".
services.AddHubtelOrdersSdk(c => {...})
is adding the Hubtel Orders SDK to the application's services, making it available for dependency injection throughout the application. The argument to AddHubtelOrdersSdk is a lambda expression that configures the SDK.
Within the lambda expression,
c.AuthType = CreateOrderAuthType.HubtelBearer
sets the authentication type to HubtelBearer. This likely means that the SDK will use bearer token authentication when making requests to the Orders API.c.AuthKey = Configuration["OrdersApiSdkConfig:AuthKey"]
sets the authentication key to the AuthKey value from the OrdersApiSdkConfig section of the application's configuration. In summary, this code configures and adds the Hubtel Orders SDK to the application's services, setting up the necessary authentication details.
services.Configure<OrdersApiSdkConfig>(Configuration.GetSection(nameof(OrdersApiSdkConfig)));
services.AddHubtelOrdersSdk(c =>
{
c.AuthType = CreateOrderAuthType.HubtelBearer;
c.AuthKey = Configuration["OrdersApiSdkConfig:AuthKey"];
}
);
Inject and consume service methods
private readonly ICreateOrderApi _orderApi
declares a private, read-only field of typeICreateOrderApi
named_orderApi
. This interface presumably provides methods for creating orders in an order processing system. The actual implementation of this interface would contain the logic for making API calls to the order processing system.var resp = await _orderApi.Create("businessId here", new CreateOrderRequest {...})
is calling theCreate
method on the_orderApi
object, which is an instance ofICreateOrderApi
. This method is asynchronous, as indicated by the await keyword, which means it will return aTask
that represents the ongoing operation to create an order.
The Create
method takes two parameters:
- A string representing the business ID. This is likely an identifier for the business that is creating the order.
- A
CreateOrderRequest
object that contains the details of the order to be created. The CreateOrderRequest object is being initialized with several properties, includingIntegrationChannel
,PaymentRequest
, and others. These properties represent various details about the order, such as the integration channel, the payment request details (including the payment channel, amount, currency, and customer details), and a callback URL.
The Create
method presumably sends a request to the order processing system to create a new order with the provided details, and returns a response that is stored in the resp
variable.
private readonly ICreateOrderApi _orderApi;
var resp = await _orderApi.Create("businessId here", new CreateOrderRequest
{
IntegrationChannel = "web-app",
PaymentRequest = new PaymentRequest
{
Channel = "momo",
PaymentProcessorRoute = "test_mtn_gh",
PaymentProcessorName = "mtn ova test",
Amount = 0.1m,
CustomerMobileNumber = "<msisdn>",
CustomerMsisdn = "<msisdn>",
SavingsAccountId = 12435,
Currency = "GHC",
PrimaryCallbackUrl = "http://localhost:3443/myurl",
CustomerName = "Augustine",
Description = "Pay me now",
}
});
Was this page helpful?
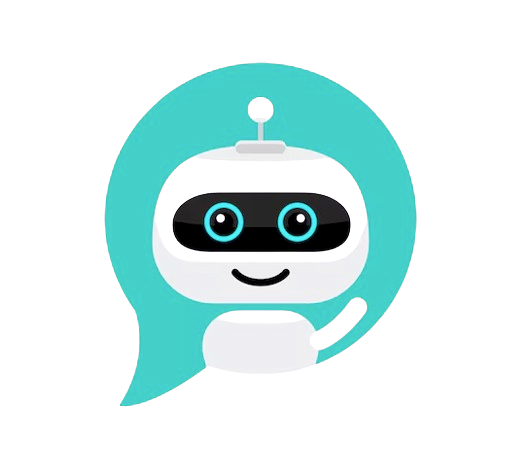
CHAT SAMMIAT