Core Network Library Documentation
Overview
The Core Network Library is a comprehensive networking solution for Flutter applications. It provides a robust set of tools and utilities for making API requests, handling responses, and managing analytics events.
Key Components
1. API Core
The ApiCore
abstract class serves as the foundation for API-related operations. It defines the basic structure for API interactions and includes methods for determining if a response has a body.
2. API Endpoint
The ApiEndPoint
class represents an API endpoint and contains all the necessary information to make a request, including the URL, request type, headers, and body.
abstract class ApiCore {
IApiRequester requester;
ApiCore({required this.requester});
bool responseHasBody({ApiResponseStatus? status}) {
if (status == ApiResponseStatus.Success) {
return true;
}
return false;
}
}
3. Data Response
The library provides ApiResponse
and DataResponse
classes to handle API responses in a structured manner.
4. Requester
The IApiRequester
interface defines the contract for making API requests. It includes methods for handling different types of requests, including multipart requests for file uploads.
5. Analytics API
The library includes components for handling analytics events:
AnalyticsApi
: Manages the posting of batch events to the analytics endpoint.EventStoreEndPoint
: Defines the endpoints for analytics events.AnalyticsApiRequester
: Handles the specifics of making requests to the analytics API.
6. Utilities
The library provides various utility functions and constants to support network operations:
ApiRequestConstants
: Contains constants used in API requests.- File compression utilities for optimizing image uploads.
Usage
Making a Simple API Request
To make an API request, you typically follow these steps:
- Create an
ApiEndPoint
object with the necessary details. - Use an implementation of
IApiRequester
to make the request. - Handle the response using the
ApiResponse
andDataResponse
classes.
Example:
var endPoint = ApiEndPoint(
address: Uri.parse('https://api.example.com/data'),
baseUrl: 'https://api.example.com',
path: '/data',
requestType: HttpVerb.GET,
body: {},
headers: {'Content-Type': 'application/json'},
);
var requester = YourApiRequesterImplementation();
var response = await requester.makeRequest(apiEndPoint: endPoint);
if (response.status == ApiResponseStatus.Success) {
// Handle successful response
} else {
// Handle error
}
Handling File Uploads
For file uploads, the library provides a makeMultipleFilesMultiPartRequest
method that supports multiple file uploads with optional compression:
var response = await makeMultipleFilesMultiPartRequest(
apiEndPoint: Future.value(ApiEndPoint(
address: Uri.parse('https://api.example.com/upload'),
files: {'images': [File('path/to/image1.jpg'), File('path/to/image2.png')]},
body: {'description': 'Multiple file upload'},
compressMedia: true,
)),
);
Posting Analytics Events
To post analytics events:
var analyticsApi = AnalyticsApi(requester: AnalyticsApiRequester());
var response = await analyticsApi.postBatchEvents(events: {
'events': [
// Your event data here
]
});
Best Practices
- Always handle potential errors and edge cases in API responses.
- Use the provided utility classes and constants to maintain consistency across your application.
- Leverage the analytics components to track important events in your application.
- When dealing with file uploads, consider using the compression feature to optimize data transfer.
Conclusion
The Core Network Library provides a robust foundation for handling network operations in Flutter applications. By leveraging its components and following the provided patterns, developers can efficiently manage API interactions, handle responses, and track analytics events.
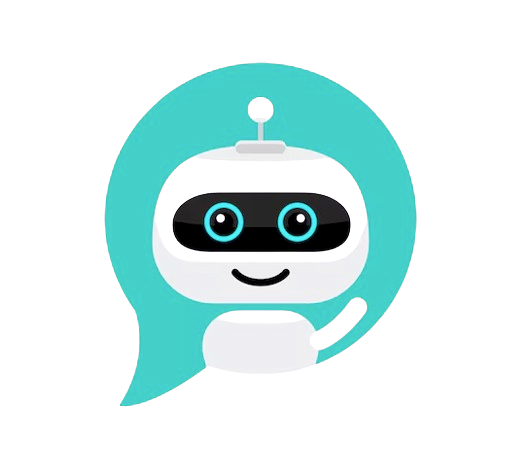
CHAT SAMMIAT