Hubtel Event Publisher: Observability Integration Guide
This guide explains how to configure and use Datadog RUM (Real User Monitoring) and Datadog browser logging with the Event Publisher to enhance application monitoring and debugging.
Setup
The configuration values will be provided by the tooling team. Ensure you consult with them to retrieve the necessary values needed for integration
Nuxt
Create a composable
useEventPublisher.ts
:tsconst eventPublisher = new HubtelEventPub({ appId: "application-id", appName: "application-name", appVersion: pkg.version, sectionName: "application-id", eventAnalyticsApiToken: ANALYTICS_EVENTS_TOKEN, sendToEventAnalytics: false, sendToEventsPortal: false, rumConfig: { applicationId: "your-datadog-application-id", clientToken: "your-datadog-client-token", service: "your-service-name", }, // logsConfig: { // override default config // } }); export default () => { return eventPublisher; };
Next
- In your
services
folder, create a fileeventPublisher.ts
// eventPublisher.ts
import { HubtelEventPub } from "@hubtel/event-publisher";
const eventPublisher = new HubtelEventPub({
appId: "application-id",
appName: "application-name",
appVersion: pkg.version,
sectionName: "application-id",
eventAnalyticsApiToken: ANALYTICS_EVENTS_TOKEN,
sendToEventAnalytics: false,
sendToEventsPortal: false,
rumConfig: {
applicationId: "your-datadog-application-id",
clientToken: "your-datadog-client-token",
service: "your-service-name",
},
// logsConfig: {
// // override default config
// }
});
export default eventPublisher;
TIP
If you wish to send events to both Event Analytics and Datadog, follow these steps:
- Set
sendToEventAnalytics
to true. - Provide a valid token to
eventAnalyticsApiToken
property.
Once configured, you can use the logAnalyticsEvent method to send events to both platforms seamlessly.
Initialisation
Next
- Create a component
Observability.tsx
to initialise datadog
// Observability.tsx
"use client";
import { eventPub } from "./eventPublisher";
eventPub.initObservability();
export default function ObservabilityInit() {
return null;
}
- Modify your root layout located under the app directory(usually app/layout.tsx) to include the ObservabilityInit component.
// app/layout.tsx
import ObservabilityInit from "./components/ObservabilityInit"; // Adjust path as necessary
export default function RootLayout({ children }) {
return (
<html lang="en">
<head />
<body>
{/* Initialize Datadog logging and RUM */}
<ObservabilityInit />
{children}
</body>
</html>
);
}
Nuxt
- Create a plugin
observabilityInit.ts
in the plugins folder:
export default defineNuxtPlugin(() => { eventPub.initObservability(); });
Usage
Logging Product Events
To facilitate a smooth migration to Datadog logging without requiring teams to change their existing method of logging events, we have integrated Datadog's logging functionality into the existinglogAnalyticsEvent
method. By setting up the datadog configuration, you can enable logging to Datadog without modifying your core event logging flow.
eventPub.logAnalyticsEvent({
eventType: "Regular",
actionName: "user_registration",
// additional properties
});
Developer Logs
The observability property exposes logging methods allowing you to log with standard log levels.
- Info
Used for logging general information about application activity. These logs indicate routine operations and successes.
eventPub.info({
eventType: "Regular",
actionName: "user_logged_in",
// additional properties
});
- Error
Logs serious issues that impact application functionality, such as runtime errors.
eventPub.error({
eventType: "Error",
actionName: "fetch_failed",
// additional properties
});
- Warn
Indicates potential issues that aren't yet critical but require attention.
eventPub.warn({
eventType: "Regular",
actionName: "restricted_feature_access",
// additional properties
});
- Debug
Logs detailed debugging information for developers to diagnose issues during development.
eventPub.debug({
eventType: "Regular",
ActionName: "Fetching user profile details",
// additional properties
});
Data Visibility on Datadog
Once Datadog RUM and logging are initialized and events are being logged, both RUM and logs data will be visible directly on Datadog’s platform. You can monitor and analyze these logs and user interactions through Datadog's web interface:
RUM Data will appear in the RUM section of Datadog, where you can view user interactions, performance metrics, and session replays. Logs Data will appear under the Logs section, where you can search, filter, and analyze logs based on various attributes, including the log levels (info, debug, warn, error), host, and custom attributes you provide.
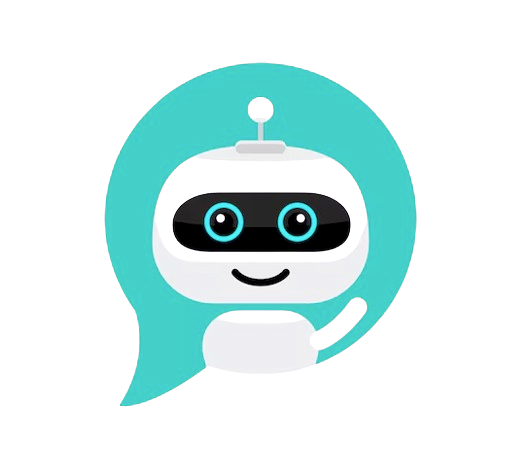
CHAT SAMMIAT